如何在 C++ 中逐行讀取檔案
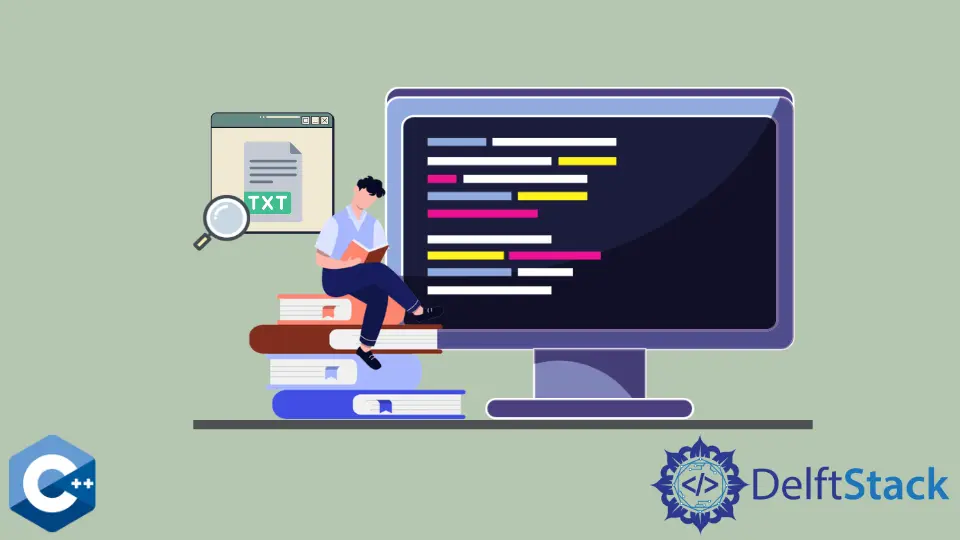
本文將介紹如何在 C++ 中逐行讀取檔案。
使用 std::getline()
函式逐行讀取檔案
getline()
函式是 C++ 中逐行讀取檔案的首選方式。該函式從輸入流中讀取字元,直到遇到定界符 char,然後將它們儲存在一個字串中。定界符作為第三個可選引數傳遞,預設情況下,它被認為是一個新的行字元/n
。
由於 getline
方法在資料可以從流中讀取的情況下會返回非零值,所以我們可以把它作為一個 while
迴圈條件,繼續從檔案中檢索行,直到到達檔案的終點。需要注意的是,最好用 is_open
方法驗證輸入流是否有關聯的檔案。
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> lines;
string line;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (getline(input_file, line)) {
lines.push_back(line);
}
for (const auto &i : lines) cout << i << endl;
input_file.close();
return EXIT_SUCCESS;
}
使用 C 庫的 getline()
函式逐行讀取一個檔案
getline
函式的使用方法類似於逐行迴圈瀏覽檔案,並將提取的行儲存在字串變數中。主要的區別是,我們應該使用 fopen
函式開啟一個檔案流,該函式返回 FILE*
物件,隨後作為第三個引數傳遞。
注意,getline
函式儲存在 char
指標,它被設定為 nullptr
。這是有效的,因為函式本身是為檢索字元動態分配記憶體的。因此,即使 getline
呼叫失敗,char
指標也應該被程式設計師明確釋放。
另外,在呼叫函式之前,將型別為 size_t
的第二個引數設定為 0 也很重要。你可以在這裡看到 getline
函式的詳細手冊。
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> lines;
char* c_line = nullptr;
size_t len = 0;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) return EXIT_FAILURE;
while ((getline(&c_line, &len, input_file)) != -1) {
lines.push_back(line.assign(c_line));
}
for (const auto& i : lines) {
cout << i;
}
cout << endl;
fclose(input_file);
free(c_line);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn