如何在 c++ 中查詢字串中的子字串
Jinku Hu
2023年10月12日
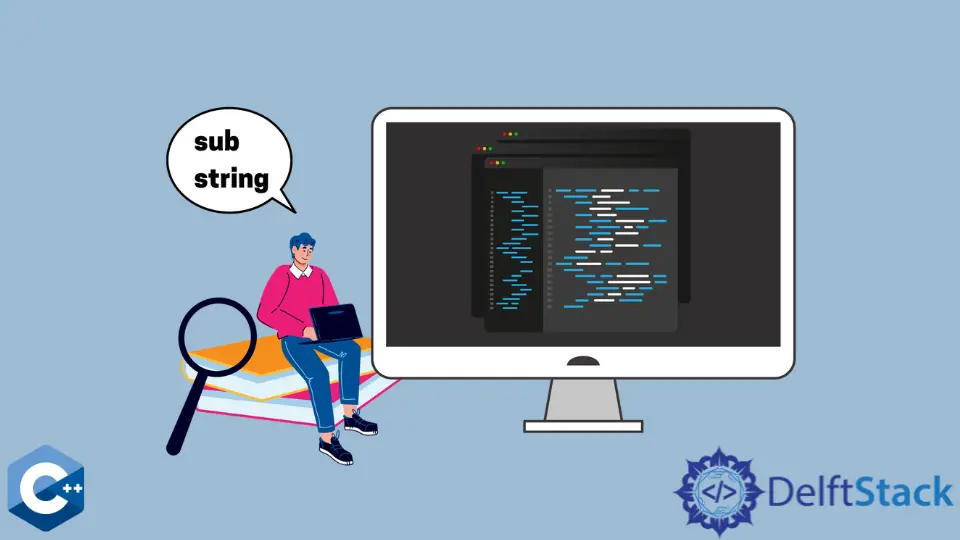
本文演示了在 C++ 中查詢字串中給定子字串的方法。
使用 find
方法在 C++ 中查詢字串中的子字串
最直接的解決方法是 find
函式,它是一個內建的字串方法,它以另一個字串物件作為引數。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
string str3 = "random s tring";
str1.find(str2) != string::npos
? cout << "str1 contains str2" << endl
: cout << "str1 does not contain str3" << endl;
str1.find(str3) != string::npos
? cout << "str1 contains str3" << endl
: cout << "str1 does not contain str3" << endl;
return EXIT_SUCCESS;
}
輸出:
str1 contains str2
str1 does not contain str3
或者,你可以使用 find
來比較兩個字串中的特定字元範圍。要做到這一點,你應該把範圍的起始位置和長度作為引數傳遞給 find
方法。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str3 = "random s tring";
constexpr int length = 6;
constexpr int pos = 0;
str1.find(str3.c_str(), pos, length) != string::npos
? cout << length << " chars match from pos " << pos << endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
輸出:
6 chars match from pos 0
使用 rfind
方法在 C++ 中查詢字串中的子字串
rfind
方法的結構與 find
類似。我們可以利用 rfind
來尋找最後的子字串,或者指定一定的範圍來匹配給定的子字串。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
str1.rfind(str2) != string::npos
? cout << "last occurrence of str3 starts at pos " << str1.rfind(str2)
<< endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
輸出:
last occurrence of str3 starts at pos 22
作者: Jinku Hu
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn