如何在 C++ 中將 Int 轉換為 ASCII 字元
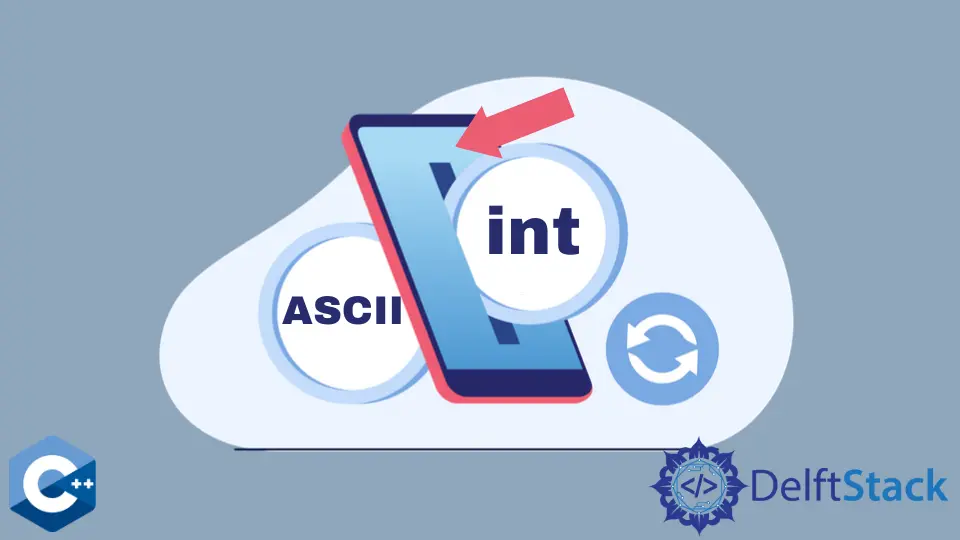
本文將為大家講解 C++ 中將整型轉為 ASCII 字元的幾種方法。
使用 Int 轉 Char 賦值將 Int 轉換為 ASCII 字元的方法
ASCII 字元編碼以 7 位格式指定。因此,有 128 個唯一的字元,每個字元都對映到 0 到 127 的相應數值。
由於 C 語言程式語言在底層實現了 char
型別的數字,所以我們可以將 int
變數賦值給 char
型別的變數,並將其視為相應的 ASCII 字元。例如,我們直接將 int
向量的值推送到 char
向量,然後使用 std::copy
方法輸出,按照預期顯示 ASCII 字元。
注意,只有當 int
值對應 ASCII 碼時,分配到 char
型別才有效,即在 0-127 範圍內。
#include <array>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
vector<char> chars{};
std::copy(numbers.begin(), numbers.end(),
std::ostream_iterator<int>(cout, "; "));
chars.reserve(numbers.size());
for (auto &number : numbers) {
chars.push_back(number);
}
cout << endl;
std::copy(chars.begin(), chars.end(),
std::ostream_iterator<char>(cout, "; "));
return EXIT_SUCCESS;
}
輸出:
97; 98; 99; 100; 101; 102; 103;
a; b; c; d; e; f; g;
或者,我們可以使用 printf
函式直接將 int
值輸出為 ASCII 字元。printf
函式對相應的型別引數採用格式指定器,如下表所示。
指定符 | 說明 |
---|---|
% |
列印%字元(這種型別不接受任何標誌、寬度、精度、長度欄位) |
d, i |
int 作為一個有符號的整數。%d 和%i 是輸出的同義詞,但當與 scanf 一起使用時,輸入就不同了(使用%i 會將數字解釋為十六進位制,如果前面是 0x,則解釋為八進位制。) |
u |
列印十進位制無符號 int |
f, F |
f 和 F 只是在無限數或 NaN 的字串列印方式上有所不同(f 為 inf、infinity 和 nan;F 為 INF、INFINITY 和 NAN) |
e,E |
標準形式的 double (d.dde±dd )。E 轉換使用字母 E(而不是 e)來引入指數。 |
g, G |
在正常或指數表示法中使用 double ,以其大小更合適。g 使用小寫字母,G 使用大寫字母。 |
x, X |
x 使用小寫字母,X 使用大寫字母。 |
o |
八進位制的無符號整型 |
s |
以空為結束的字串 |
c |
char(字元) |
p |
void* (指向 void 的指標),採用執行定義的格式。 |
a, A |
以十六進位制表示法的 double 值,從 0x 或 0X 開始。a 使用小寫字母,A 使用大寫字母。 |
n |
不列印任何內容,但將目前成功寫入的字元數寫入一個整數指標引數中。 |
#include <array>
#include <charconv>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
vector<char> chars{};
for (const auto &number : numbers) {
printf("%c; ", number);
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
a; b; c; d; e; f; g;
使用 sprintf()
函式將 Int 轉換為 ASCII 字元
sprintf
函式是另一種將 int
值轉換為字元的方法。我們必須宣告一個 char
變數來儲存轉換後的值。這個變數作為 sprintf
的第一個引數傳遞給 sprintf
,第三個引數指定要處理的數字。最後,你應該像 printf
一樣提供一個格式指定符,它表示值將被轉換為哪種型別。
#include <array>
#include <charconv>
#include <iostream>
#include <iterator>
#include <vector>
using std::array;
using std::copy;
using std::cout;
using std::endl;
using std::to_chars;
using std::vector;
int main() {
vector<int> numbers{97, 98, 99, 100, 101, 102, 103};
array<char, 5> char_arr{};
for (auto &number : numbers) {
sprintf(char_arr.data(), "%c", number);
printf("%s; ", char_arr.data());
}
cout << endl;
return EXIT_SUCCESS;
}
a; b; c; d; e; f; g;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn