在 C++ 中的向量中查詢元素索引
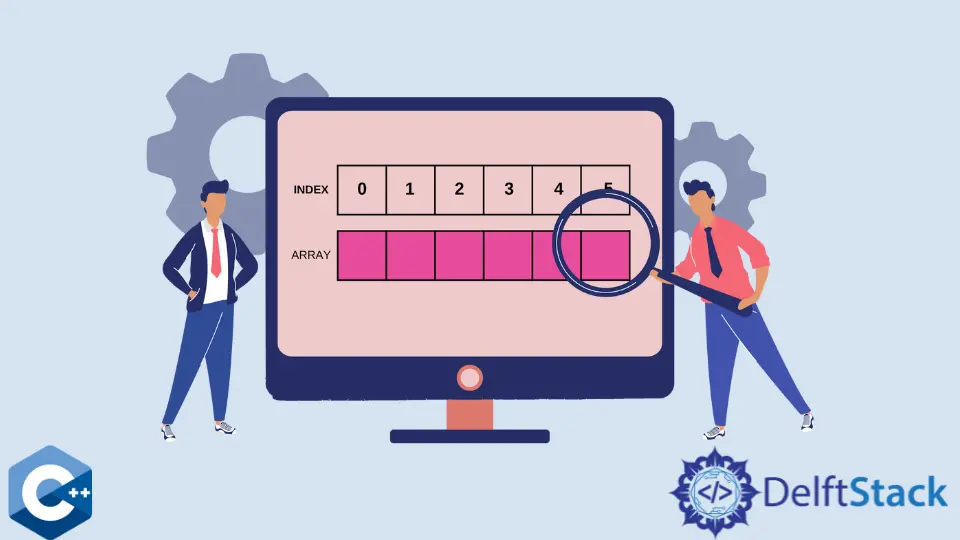
本文將介紹幾種在 C++ 中如何在向量中查詢元素索引的方法。
在 C++ 中使用自定義函式查詢向量中的元素索引
我們可以使用自定義線性搜尋功能在 vector
中找到給定元素的位置。請注意,這是解決此問題的一種非常無效的方法。在下面的示例程式碼中,我們宣告一個整數的向量,並尋找一個任意的元素位置,如果呼叫成功,則將其輸出。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex(const vector<int> &arr, int item) {
for (auto i = 0; i < arr.size(); ++i) {
if (arr[i] == item) return i;
}
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos = findIndex(arr, 32);
pos != -1
? cout << "Found the element " << 32 << " at position " << pos << endl
: cout << "Could not found the element " << 32 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
輸出:
Could not found the element 32 in vector
在 C++ 中使用 std::find
演算法查詢向量中的元素索引
另外,我們可以使用 STL 庫中的 std::find
演算法。此函式將迭代器返回到滿足條件的第一個元素。另一方面,如果未找到任何元素,則演算法將返回範圍的最後一個元素。請注意,返回的迭代器應按 begin
迭代器遞減以計算位置。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int findIndex2(const vector<int> &arr, int item) {
auto ret = std::find(arr.begin(), arr.end(), item);
if (ret != arr.end()) return ret - arr.begin();
return -1;
}
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto pos2 = findIndex2(arr, 10);
pos2 != -1
? cout << "Found the element " << 10 << " at position " << pos2 << endl
: cout << "Could not found the element " << 10 << " in vector" << endl;
exit(EXIT_SUCCESS);
}
輸出:
Found the element 10 at position 9
在 C++ 中使用 std::find_if
演算法查詢向量中的元素索引
查詢元素索引的另一種方法是呼叫 std::find_if
演算法。它與 std::find
相似,除了第三個引數可以是用於評估每個迭代元素的謂詞表示式。如果表示式返回 true,則演算法將返回。注意,我們將 lambda 表示式作為第三個引數傳遞,該表示式檢查元素是否等於 10
。
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main(int argc, char *argv[]) {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto ret =
std::find_if(arr.begin(), arr.end(), [](int x) { return x == 10; });
if (ret != arr.end())
cout << "Found the element " << 10 << " at position " << ret - arr.begin()
<< endl;
exit(EXIT_SUCCESS);
}
輸出:
Found the element 10 at position 9
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn