在 C++ 中生成斐波那契數
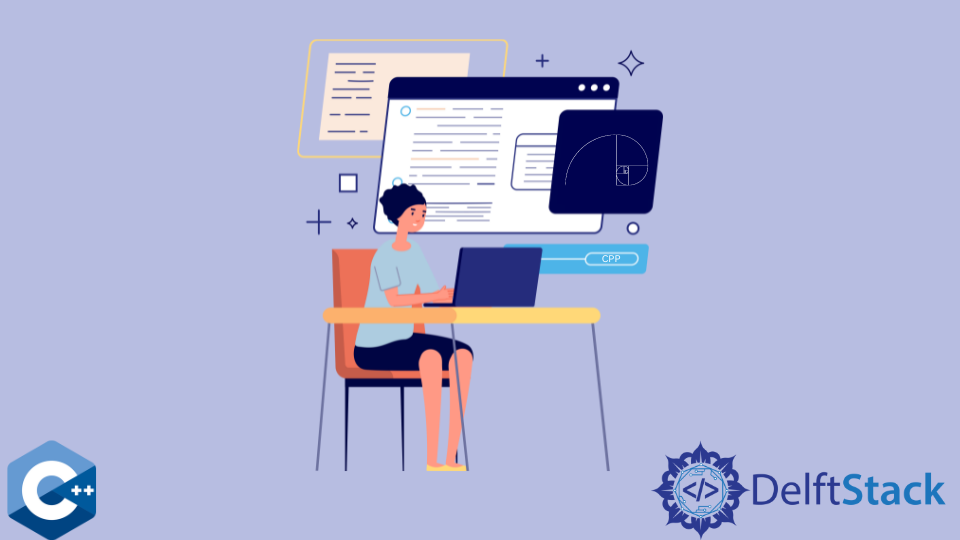
本文將演示如何在 C++ 中生成斐波那契數的多種方法。
C++ 使用迭代方法來列印斐波那契數列中的前 n
項
斐波那契數在數學上通常被稱為數字序列,其中每個數字都是從 0
和 1
開頭的前兩數字之和。在此示例程式碼中,我們將使用者輸入作為整數 n
並生成斐波那契數列中的前 n
數字。解決方案是簡單的迭代,在此我們初始化起始值並迭代 n-3
次以求和先前的值。每次迭代,總和被列印到 cout
流中,並且儲存的值被移動一個數字視窗。注意,實現了 if
條件,該條件檢查輸入整數 0
並列印相應的值。第一個 cout
語句處理 n
等於 2
時的情況,因為 for
迴圈條件的結果為 false
,而不會繼續。
#include <iostream>
using std::cout; using std::cin;
using std::endl; using std::string;
void generateFibonacci(unsigned long long n)
{
if (n == 1) {
cout << 0 << endl;
return;
}
unsigned long long a = 0;
unsigned long long b = 1;
unsigned long long c;
cout << a << " " << b;
for (unsigned long long i = 3; i <= n; i++)
{
c = a + b;
cout << " " << c;
a = b;
b = c;
}
}
int main()
{
unsigned long long num;
cout << "Enter the number of items to generate Fibonacci series: ";
cin >> num;
generateFibonacci(num);
return EXIT_SUCCESS;
}
輸出:
Enter the number of items to generate Fibonacci series: 10
0 1 1 2 3 5 8 13 21 34
C++ 使用迭代方法列印斐波那契數列中的第 n 個項
另外,我們可以實現一個函式,該函式返回斐波那契數列中的第 n 個數字。注意,我們將使用者輸入儲存在 unsigned long long
中,因為我們希望能夠輸出內建 C++ 型別可以儲存的最大數字。該函式以 if-else
語句開始,以檢查前三種情況並返回硬編碼值。另一方面,如果 n
大於 3
,我們將迴圈迴圈並迭代直到獲得序列中的第 n 個數字。
#include <iostream>
using std::cout; using std::cin;
using std::endl; using std::string;
unsigned long long generateFibonacci(unsigned long long n)
{
if (n == 1) {
return 0;
} else if (n == 2 || n == 3) {
return 1;
}
unsigned long long a = 1;
unsigned long long b = 1;
unsigned long long c;
for (unsigned long long i = 3; i < n; i++)
{
c = a + b;
a = b;
b = c;
}
return c;
}
int main()
{
unsigned long long num;
cout << "Enter the n-th number in Fibonacci series: ";
cin >> num;
cout << generateFibonacci(num);
cout << endl;
return EXIT_SUCCESS;
}
輸出:
Enter the n-th number in Fibonacci series: 10
34
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn