在 C++ 中使用波浪操作符來定義類解構函式
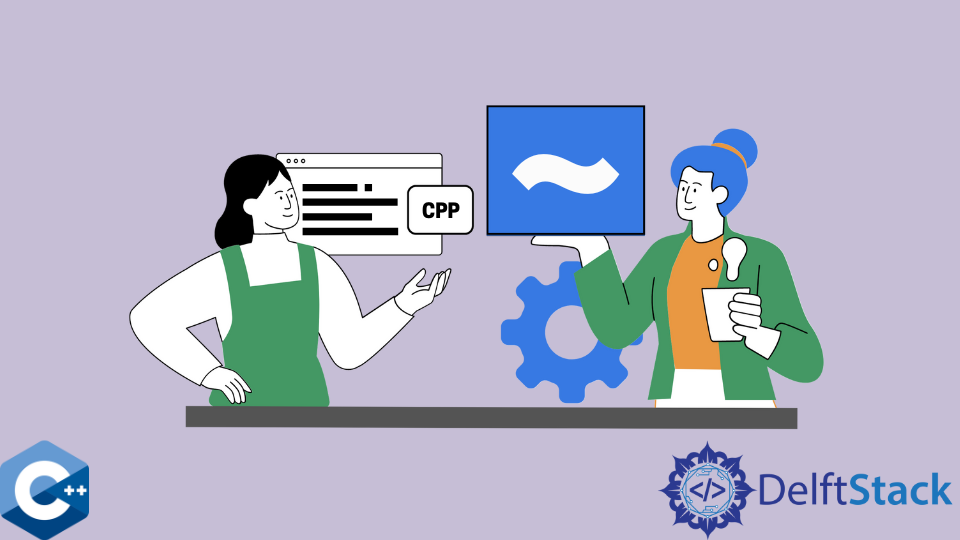
本文將演示多種方法,介紹如何在 C++ 中使用波浪操作符定義類的解構函式。
在 C++ 中使用波浪符號~
來宣告類的解構函式
解構函式是一個特殊的成員函式,它處理類物件資源的釋放,與類建構函式相比,它對特定的類只有一個解構函式。與類建構函式相反,一個類只有一個解構函式。類的解構函式是用與類相同的名稱加上字首~
波浪運算子來宣告的。
在大多數情況下,如果有資料成員需要在動態記憶體上分配,則需要定義類的解構函式。程式碼應該在解構函式中顯式地釋放這些成員。下面的例子演示了 CustomString
類,它只是 std::string
的包裝器。我們的想法是定義一個類,其建構函式在 str
指標處分配記憶體,這意味著該類需要定義解構函式。注意,CustomString
有兩個建構函式-其中一個是複製建構函式。
#include <iostream>
#include <string>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::string;
class CustomString {
public:
explicit CustomString(const string &s = string()):
str(new string(s)) { }
CustomString(const CustomString &p):
str(new string(*p.str)) { }
~CustomString() { delete str; }
string& getString() { return *str; };
private:
string *str;
};
int main()
{
CustomString str1("Hello There!");
CustomString str2(str1);
cout << "str1: " << str1.getString() << endl;
cout << "str2: " << str2.getString() << endl << endl;
}
輸出:
str1: Hello There!
str2: Hello There!
在 C++ 中銷燬類物件之前執行程式碼
就像前面的方法所示,類的解構函式負責清理資料成員的記憶體。雖然,類成員往往只是在程式棧上宣告的普通資料型別。在這種情況下,解構函式可能不會被程式設計師明確宣告,而是由編譯器定義所謂的合成解構函式。
一般來說,類成員是在解構函式程式碼執行後被銷燬的,因此,我們可以演示一下 StringArray
類例項是如何跳出範圍的,從而向控制檯列印相應的文字。
#include <iostream>
#include <string>
#include <vector>
using std::cout; using std::endl;
using std::vector; using std::string;
class StringArray {
public:
StringArray(): vec(), init(new int[10]) { };
~StringArray() { delete [] init; cout << "printed from destructor" << endl; }
string getString(int num) {
if (vec.at(num).c_str()) {
return vec.at(num);
} else {
return string("NULL");
}
};
void addString(const string& s) {
vec.push_back(s);
};
uint64_t getSize() { return vec.size(); };
private:
vector<string> vec;
int *init;
};
int main()
{
StringArray arr;
arr.addString("Hello there 1");
arr.addString("Hello there 2");
cout << "size of arr: " << arr.getSize() << endl;
cout << "arr[0]: " << arr.getString(0) << endl;
}
輸出:
size of arr: 2
arr[0]: Hello there 1
printed from destructor
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn