在 C++ 中檢查字串是否為迴文
-
使用
string
複製建構函式與rbegin
/rend
方法來檢查 C++ 中的字串是否為迴文 -
使用
std::equal
方法在 C++ 中檢查字串迴文 - 使用自定義函式在 C++ 中檢查字串是否是迴文
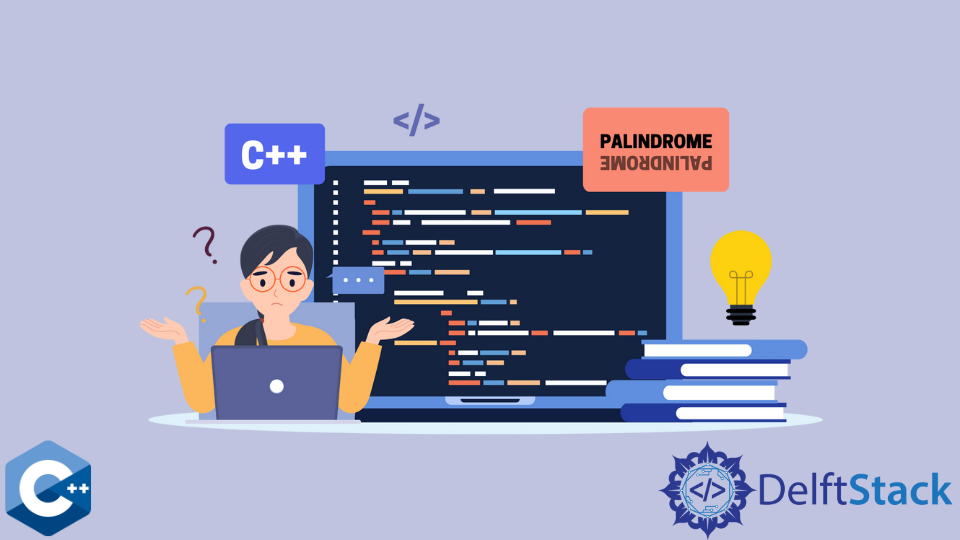
本文將為大家講解幾種在 C++ 中如何檢查一個字串是否為迴文的方法。
使用 string
複製建構函式與 rbegin
/rend
方法來檢查 C++ 中的字串是否為迴文
string
類物件支援使用運算子 ==
進行比較,它可以用來尋找符合迴文模式的字串。由於迴文意味著按相反的順序匹配字元,我們需要用 rbegin
和 rend
迭代器構造一個新的字串物件。其餘的則根據需要在 if
語句中構造。
在下面的例子中,我們宣告瞭兩個字串 - 單字迴文和多字迴文。請注意,儘管帶空格的字串符合定義模式,但它不能將帶有空格的字串作為迴文式檢測。
#include <iostream>
#include <string>
using std::cout; using std::endl;
using std::string; using std::cin;
using std::equal; using std::remove;
int main(){
string s1 = "radar";
string s2 = "Was it a cat I saw";
if (s1 == string(s1.rbegin(), s1.rend())) {
cout << "s1 is a palindrome" << endl;
} else {
cout << "s1 is not a palindrome" << endl;
}
if (s2 == string(s2.rbegin(), s2.rend())) {
cout << "s2 is a palindrome" << endl;
} else {
cout << "s2 is not a palindrome" << endl;
}
return EXIT_SUCCESS;
}
輸出:
s1 is a palindrome
s2 is not a palindrome
使用 std::equal
方法在 C++ 中檢查字串迴文
儘管最後一個實現在單字字串上完成了工作,但它帶來了建立一個物件副本和比較它們的完整範圍的開銷。我們可以利用 std::equal
演算法來比較同一個 string
物件範圍的前半部分和後半部分。std::equal
如果給定的兩個範圍中的元素相等,則返回布林值 true
。請注意,函式只需要一個迭代器-s1.rbegin()
用於第二個範圍,因為範圍的結束是以 first2 + (last1 - first1)
計算的。
#include <iostream>
#include <string>
using std::cout; using std::endl;
using std::string; using std::cin;
using std::equal; using std::remove;
int main(){
string s1 = "radar";
string s2 = "Was it a cat I saw";
equal(s1.begin(), s1.begin() + s1.size()/2, s1.rbegin()) ?
cout << "s1 is a palindrome" << endl :
cout << "s1 is not a palindrome" << endl;
equal(s2.begin(), s2.begin() + s2.size()/2, s2.rbegin()) ?
cout << "s2 is a palindrome" << endl :
cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
輸出:
s1 is a palindrome
s2 is not a palindrome
使用自定義函式在 C++ 中檢查字串是否是迴文
以前的方法對多字的字串有不足之處,我們可以通過實現一個自定義函式來解決。本例演示了布林函式 checkPalindrome
,它接受 string&
引數,並將其值儲存在本地 string
變數中。然後用 transform
演算法處理本地物件,將其轉換為小寫字母,並因此用 eras-remove
刪除其中的所有空白字元。最後,我們在 if
語句條件中呼叫 equal
演算法,並返回相應的布林值。不過要注意的是,如果字串是由多位元組字元組成的,這個方法會失敗。因此,應該實現支援所有通用字元編碼方案的小寫轉換方法。
#include <iostream>
#include <string>
using std::cout; using std::endl;
using std::string; using std::cin;
using std::equal; using std::remove;
bool checkPalindrome(string& s) {
string tmp = s;
transform(tmp.begin(), tmp.end(), tmp.begin(),
[](unsigned char c){ return tolower(c); } );
tmp.erase(remove(tmp.begin(), tmp.end(), ' '), tmp.end());
if (equal(tmp.begin(), tmp.begin() + tmp.size()/2, tmp.rbegin())) {
return true;
} else {
return false;
}
}
int main(){
string s1 = "radar";
string s2 = "Was it a cat I saw";
checkPalindrome(s1) ?
cout << "s1 is a palindrome" << endl :
cout << "s1 is not a palindrome" << endl;
checkPalindrome(s2) ?
cout << "s2 is a palindrome" << endl :
cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
輸出:
s1 is a palindrome
s2 is a palindrome
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn