從 C++ 中的字串中獲取最後一個字元
Suraj P
2023年1月30日
2022年4月20日
-
在 C++ 中使用
std::string::at
從字串中獲取最後一個字元 -
在 C++ 中使用
std::string::back()
從字串中獲取最後一個字元 -
使用
std::string::rbegin()
從 C++ 中的字串中獲取最後一個字元 -
在 C++ 中使用
std::string:begin()
和reverse()
從字串中獲取最後一個字元 - 在 C++ 中使用陣列索引訪問字串的字元
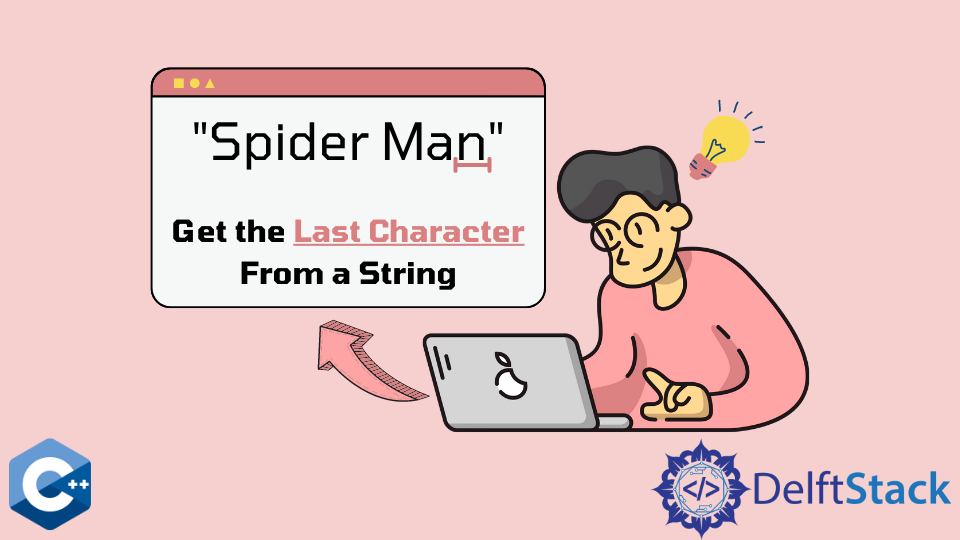
本文將教授 C++ 中從字串中獲取最後一個字元的不同方法。
在 C++ 中使用 std::string::at
從字串中獲取最後一個字元
我們可以使用 std::string::at
從字串中提取出現在給定位置的字元。
語法:
char& string::at(size_type indexNo)
這將返回使用者指定的 indexNo
處指定位置的字元。當傳遞了無效的索引號時,該方法會丟擲 out_of_range
異常,例如小於零的索引或大於或等於 string.size()
的索引。
示例:提取最後一個字元和給定位置的字元。
#include <iostream>
using namespace std;
int main()
{
string str("Spider Man");
cout<<"Character at position 3: "<<str.at(3)<<endl;
int length = str.size();
cout<<"Character at last position: "<<str.at(length-1)<<endl;
}
解釋
為了得到最後一個位置的字元,我們首先找到字串的長度,並在
std::string::at()
方法中傳遞 length-1
。輸出:
Character at position 3: d
Character at last position: n
在 C++ 中使用 std::string::back()
從字串中獲取最後一個字元
C++ 中的 std::string::back()
給出了對字串最後一個字元的引用。這僅適用於非空字串。
此函式還可以替換字串末尾的字元。
語法:
- 訪問最後一個字元。
char ch = string_name.back();
- 替換出現在字串末尾的字元。
str.back() = 'new_char_we_to_replace_with';
此函式不帶引數,它提供對字串最後一個字元的引用。當應用於空字串時,它會顯示未定義的行為。
示例程式碼:
#include <iostream>
using namespace std;
int main()
{
string str("Spider Man");
char ch = str.back();
cout<<"Character at the end "<<ch<<endl;
str.back() = '@';
cout<<"String after replacing the last character: "<<str<<endl;
}
輸出:
Character at the end n
String after replacing the last character: Spider Ma@
使用 std::string::rbegin()
從 C++ 中的字串中獲取最後一個字元
C++ 中的 std::string::rbegin()
代表反向開始。該函式用於指向字串的最後一個字元。
語法:
reverse_iterator it = string_name.rbegin();
該函式不包含任何引數,它返回指向字串最後一個字元的反向迭代器。
示例程式碼:
#include <iostream>
#include<string>
using namespace std;
int main()
{
string str("Spider Man");
string::reverse_iterator it = str.rbegin();
cout<<"The last character of the string is: "<<*it;
}
輸出:
The last character of the string is: n
在 C++ 中使用 std::string:begin()
和 reverse()
從字串中獲取最後一個字元
C++ 中的 std::string::begin()
將迭代器返回到字串的開頭,不帶引數。
語法:
string::iterator it = string_name.begin();
std::string::reverse()
是 C++ 中用於直接反轉字串的內建函式。它將 begin
和 end
迭代器作為引數。
該函式在 algorithm
標頭檔案中定義。
語法:
reverse(string_name.begin(), string_name.end());
要從字串中獲取最後一個字元,我們首先使用 reverse
方法反轉字串,然後使用 begin()
方法獲取字串第一個字元的引用(這是反轉之前的最後一個字元)並列印它。
示例程式碼:
#include <iostream>
#include<string>
#include<algorithm>
using namespace std;
int main()
{
string str("Spider Man");
reverse(str.begin(),str.end());
string::iterator it = str.begin();
cout<<"The last character of the string is: "<<*it;
}
輸出:
The last character of the string is: n
在 C++ 中使用陣列索引訪問字串的字元
我們可以通過使用它的索引號引用它並在方括號 []
中傳遞它來訪問字串的字元。
示例程式碼:
#include <iostream>
using namespace std;
int main()
{
string str("Bruce banner");
int length = str.size();
cout<<"The last character of the string is "<<str[length-1];
}
為了得到最後一個字元,我們首先找到字串的長度並在方括號中傳遞 length-1
。
輸出:
The last character of the string is r
Author: Suraj P