用 C++ 驗證使用者輸入
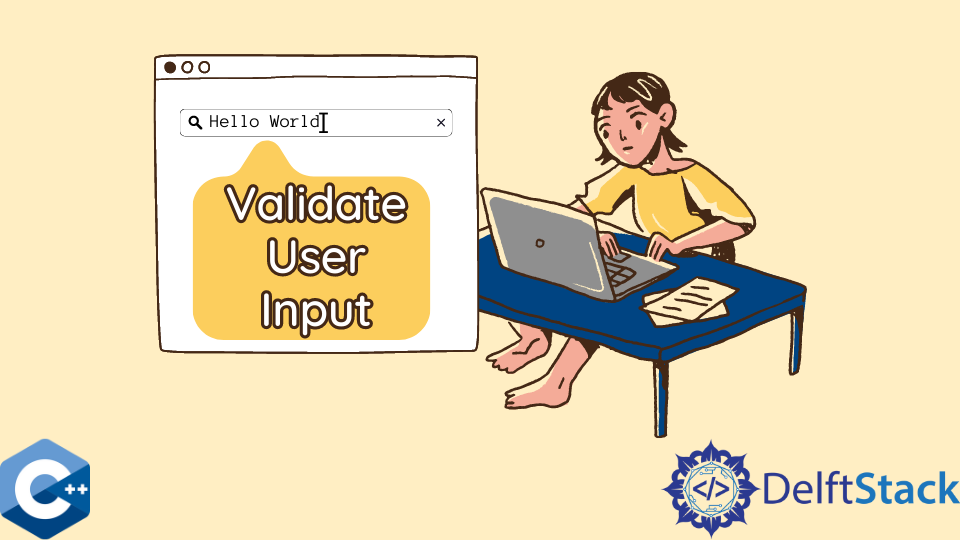
本文將演示關於如何在 C++ 中驗證使用者輸入的多種方法。
使用 cin
與 cin.clear
和 cin.ignore
方法來驗證使用者輸入
這個例子的重點是一個健壯的使用者輸入驗證方法,這意味著它應該滿足基本的錯誤檢查要求,即使輸入的型別是錯誤的,也要繼續執行。
實現這個功能的第一個構造是 while
迴圈,我們要在一個條件中指定 true
。這個迭代將保證迴圈行為,直到正確的值被儲存在一個變數中。在迴圈內部,一個 if
語句會評估 cin >> var
表示式,因為插入操作成功後,返回值為正。一旦正確地儲存了值,我們就可以退出迴圈;否則,如果 cin
表示式沒有成功,執行就會進入 cin.clear
呼叫,在意外輸入後取消設定 failbit
。接下來,我們跳過輸入緩衝區中剩餘的字元,進入下一次迭代詢問使用者。
#include <iostream>
#include <sstream>
#include <limits>
#include <vector>
using std::string; using std::vector;
using std::cout; using std::endl;
using std::cin; using std::numeric_limits;
int main()
{
int age;
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> age) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return EXIT_SUCCESS;
}
輸出:
Enter the age of the wine: 32
使用自定義函式來驗證使用者的輸入
以前的方法即使對於幾個輸入變數也是相當麻煩的,而且多個 while
迴圈會浪費程式碼空間。因此,應將子程式概括為一個函式。validateInput
是函式模板,它接收變數的引用並返回成功儲存的值。
注意,即使我們需要實現一個 100 道題的測驗,這個方法也能保證比之前的版本產生的程式碼乾淨得多。
#include <iostream>
#include <sstream>
#include <limits>
#include <vector>
using std::string; using std::vector;
using std::cout; using std::endl;
using std::cin; using std::numeric_limits;
template<typename T>
T &validateInput(T &val)
{
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> val) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main()
{
int age;
int volume;
int price;
age = validateInput(age);
volume = validateInput(volume);
price = validateInput(price);
cout << "\nYour order's in!\n" << age << " years old Cabernet"
<< " in " << volume << "ml bottle\nPrice: " << price << "$\n";
return EXIT_SUCCESS;
}
輸出:
Enter the age of the wine: 13
Enter the volume of the wine in milliliters: 450
Enter the price of the bottle: 149
Your order's in!
13 years old Cabernet in 450ml bottle
Price: 149$
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn