在 C++ 中新增定時延遲
-
在 C++ 中使用
sleep()
系統呼叫新增定時延遲 -
在 C++ 中使用
usleep()
函式新增定時延遲 -
使用
sleep_for()
函式在 C++ 中新增定時延遲 -
在 C++ 中使用
sleep_until()
函式新增定時延遲
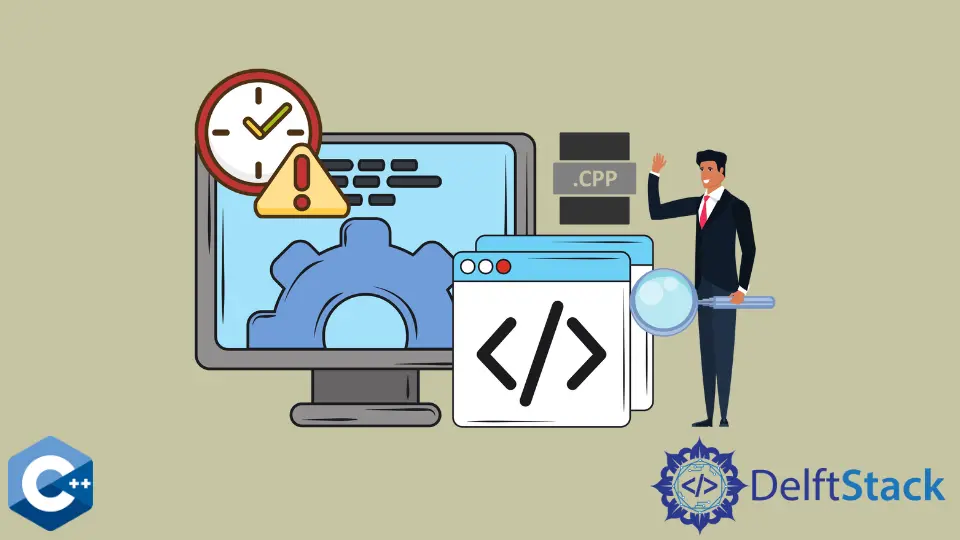
本教程將為你提供有關在 C++ 程式中新增定時延遲的簡要指南。
這可以使用 C++ 庫為我們提供的一些函式以多種方式完成。我們將討論一些功能及其工作演示。
計算機程式中的任何程序、任務或執行緒都可以休眠或變為非活動狀態。這意味著程序的執行可以在它處於睡眠狀態時暫停。
當休眠間隔期滿或訊號或中斷導致執行恢復時,將恢復執行。為此目的有不同的方法:
sleep()
系統呼叫usleep()
方法sleep_for()
方法sleep_until()
方法
在 C++ 中使用 sleep()
系統呼叫新增定時延遲
sleep
系統呼叫可以使程式(任務、程序或執行緒)進入睡眠狀態。典型的 sleep
系統呼叫中的 time
參數列明程式需要睡眠或保持非活動狀態的時間。
C++ 語言沒有睡眠
功能。此功能由特定於作業系統的檔案提供,例如 Linux 的 unistd.h
和 Windows 的 Windows.h
。
要在 Linux 或 UNIX 作業系統上使用 sleep()
函式,我們必須在程式中包含 "unistd.h"
標頭檔案。
函式原型
unsigned sleep(unsigned seconds);
它需要我們需要暫停執行的引數秒數,如果成功返回執行,則返回 0。如果睡眠在兩者之間被中斷,則返回總時間減去中斷時間。
例子:
#ifdef _WIN32
#include <Windows.h>
#else
#include <unistd.h>
#endif
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
cout << "Before sleep call " << endl;
cout.flush();
sleep(9);
cout << "After sleep call" << endl;
return 0;
}
輸出:
Before sleep call
After sleep call
在輸出中,我們列印了兩條語句。列印第一個後,編譯器等待 9 秒,然後列印另一個。讀者應該執行程式來觀察這個延遲。
在 C++ 中使用 usleep()
函式新增定時延遲
標頭檔案 unistd.h
中的另一個函式是 usleep()
,它允許你暫停程式的執行一段時間。該操作與前面描述的 sleep()
函式相同。
函式 usleep()
將呼叫執行緒的執行暫停 useconds microseconds
或直到傳送中斷執行的訊號。
函式原型
int usleep(useconds_t useconds);
此函式將需要暫停執行的微秒數作為引數,如果成功恢復,則返回 0,如果發生故障,則返回 -1。
例子:
#include <unistd.h>
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
cout << "Hello ";
cout.flush();
usleep(10000);
cout << "World";
cout << endl;
return 0;
}
輸出:
Before Usleep Call
After Usleep call
正如你在上面的程式碼片段中所看到的,我們在列印兩個語句之間提供了 10000 微秒的等待時間。
在 C++ 11 中,我們提供了使執行緒休眠的特定函式。為此目的有兩種方法,sleep_for
和 sleep_until
。
讓我們討論這兩種方法:
使用 sleep_for()
函式在 C++ 中新增定時延遲
sleep_for ()
函式在 <thread>
標頭檔案中定義。sleep_for ()
函式在睡眠持續時間中指定的持續時間內暫停當前執行緒的執行。
由於排程活動或資源爭用延遲,此功能可能會阻塞超過提供的時間段。
函式原型
template <class Rep, class Period>
void sleep_for(const std::chrono::duration<Rep, Period>& sleep_duration);
例子:
#include <chrono>
#include <iostream>
#include <thread>
using namespace std;
int main() {
cout << "Hello I'm here before waiting" << endl;
this_thread::sleep_for(chrono::milliseconds(10000));
cout << "I Waited for 10000 ms\n";
}
輸出:
Hello I'm here before waiting
I Waited for 10000 ms
在上面的程式碼片段中,我們讓主執行緒休眠 10000 毫秒,這意味著當前執行緒將阻塞 10000 毫秒,然後恢復執行。
在 C++ 中使用 sleep_until()
函式新增定時延遲
<thread>
標頭檔案定義了這個函式。sleep_until ()
方法停止執行緒執行,直到經過睡眠時間。
由於排程活動或資源爭用延遲,此功能與其他功能一樣,可能會阻塞超過指定時間。
函式原型
template <class Clock, class Duration>
void sleep_until(const std::chrono::time_point<Clock, Duration>& sleep_time);
它將需要阻塞執行緒的週期作為引數,並且不返回任何內容。
例子:
#include <chrono>
#include <iostream>
#include <thread>
using namespace std;
int main() {
cout << "Hello I'm here before waiting" << endl;
std::this_thread::sleep_until(std::chrono::system_clock::now()
std::chrono::seconds(3));
cout << "I Waited for 3 seconds\n";
}
輸出:
Hello I'm here before waiting
I Waited for 2 seconds
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn