在 C++ 中檢查字串是否為空
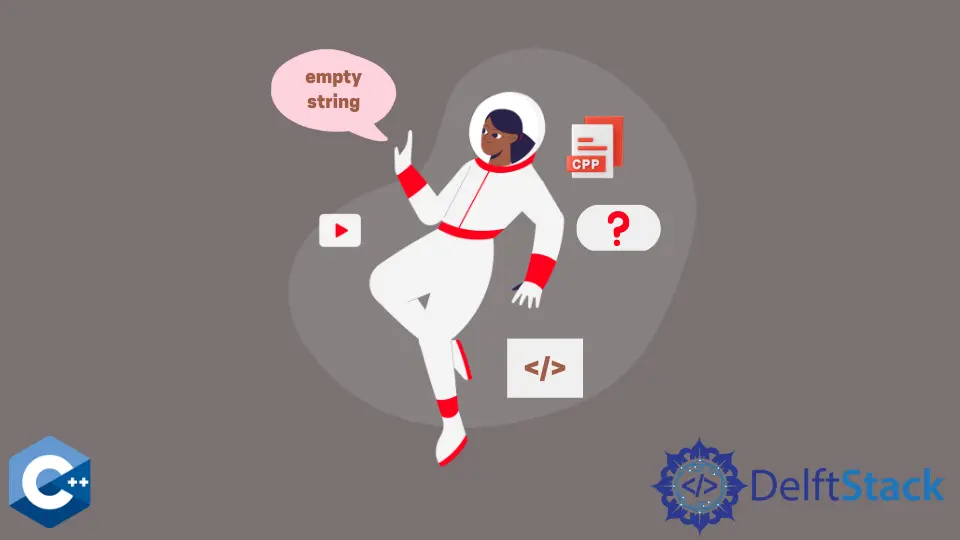
本文將介紹關於如何在 C++ 中檢查空字串的多種方法。
使用內建方法 empty()
來檢查 C++ 中的字串是否為空
std::string
類有一個內建的方法 empty()
來檢查給定的字串是否為空。這個方法的型別是 bool
,當物件不包含字元時返回 true
。empty()
方法不接受任何引數,並實現了一個恆時複雜度函式。
請注意,即使字串被初始化為空字串字面–""
,該函式仍然返回 true
值。因此,empty()
函式檢查字串的大小,並本質上返回表示式的評估值-string.size() == 0
。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string string1("This is a non-empty string");
string string2;
string1.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
string2.empty() ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
輸出:
string value: This is a non-empty string
[ERROR] string is empty!
在 C++ 中使用自定義定義的 size
函式檢查字串是否為空
前一種方法可以由使用者定義的函式來實現,該函式接受一個 string
引數並檢查其是否為空。這個函式將反映 empty
方法的行為,並返回一個 bool
值。下面的例子演示了使用自定義函式 checkEmptyString
的相同程式碼示例。請注意,該函式只包含一條語句,因為比較表示式的返回型別將是布林值,我們可以直接將其傳遞給 return
關鍵字。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const string &s) { return s.size() == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2) ? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
輸出:
string value: This is a non-empty string
[ERROR] string is empty!
使用 strlen()
函式檢查 C++ 中的字串是否為空
strlen()
函式是 C 字串庫的一部分,可以用來檢索字串的位元組大小。對於程式碼庫中可能出現的 string
和 char*
型別的字串,這種方法可以更加靈活。strlen
接受 const char*
引數,並計算出不包括終止符 \0
的長度。
請注意,該程式的結構與前面的方法類似,因為我們定義了一個單獨的布林函式,並將其唯一的引數宣告為 const char*
。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool checkEmptyString(const char *s) { return strlen(s) == 0; }
int main() {
string string1("This is a non-empty string");
string string2;
checkEmptyString(string1.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
checkEmptyString(string2.data())
? cout << "[ERROR] string is empty!" << endl
: cout << "string value: " << string1 << endl;
return EXIT_SUCCESS;
}
輸出:
string value: This is a non-empty string
[ERROR] string is empty!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn