C++ 中字串和字元的比較
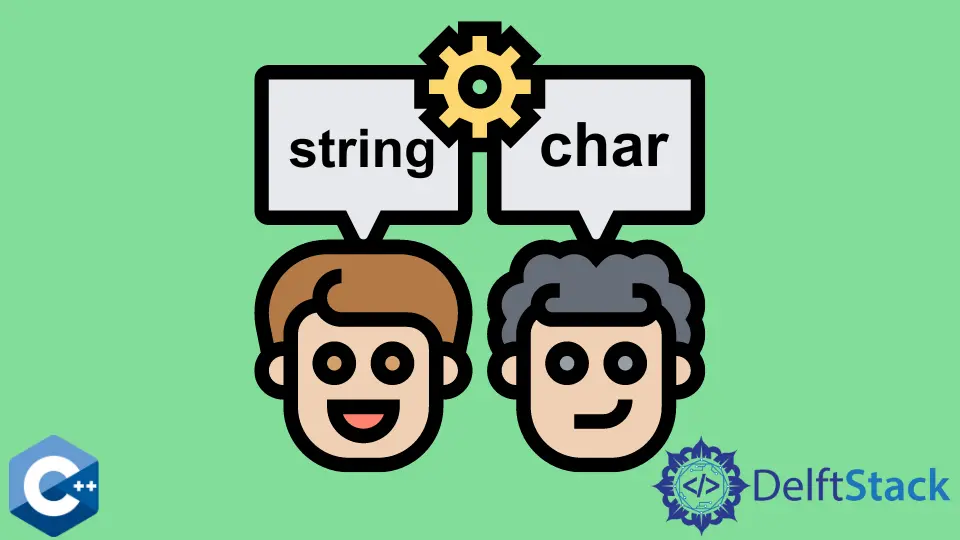
這個簡單的指南是關於在 C++ 中使用字串以及如何將這些字串與其他文字進行比較。在繼續之前,我們將簡要介紹 C++ 中的字串。
在 C++ 中,字串可以按兩種不同的方式分類:
- 建立一個
Character
陣列以形成一個字串 - 在 C++ 中使用標準的
String
庫
在 C++ 中建立字元陣列
與 C 語言一樣,C++ 也為我們提供了字元陣列,即 char
陣列,它可以用作字串文字。它是一個以 null
結尾的一維字元陣列。
因此,字串是通過形成一個 char
陣列並以 null
字元終止它來建立的。
請注意,要使用聚合方法(例如,cout<< charArray
)列印字元陣列,null
字元必須終止字元陣列。C++ 使用 \0
作為 NULL
字元,它有助於聚合方法來檢測字串的結尾(如果沒有一些結束標記符號就不可能檢測到陣列的結尾)。
因此,下面的示例宣告瞭一個大小為 11
的字元陣列,儘管 C 語言
僅包含十個字元。
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
上面的語句也可以寫成下面這樣,前提是你遵循初始化規則。
char word[] = "C-language";
如果你忘記將 null
字元放在末尾,編譯器會隱式地將 null
字元放在末尾。讓我們看看下面的程式。
#include <iostream>
using namespace std;
int main() {
char word[11] = {'C', '-', 'l', 'a', 'n', 'g', 'u', 'a', 'g', 'e', '\0'};
cout << "First message: ";
cout << word << endl;
return 0;
}
上述程式碼片段中的第 06 行將向輸出提供 First Message:
,而第 07 行將顯示 word
變數中的所有字元,直到遇到\0
。因此,上面的程式碼片段將生成以下輸出。
First Message: C-language
在 C++ 中使用 String
庫
C++ 有一個內建的 string.h
標頭檔案作為其標準庫的一部分。它提供了一系列功能(例如,strcpy
、strlen
等)來處理 C 風格的字串(即,以 null 結尾的字串)。
請注意,所有沒有 .h
的現代 string
庫都與 string.h
不同。string
類庫 是一個用於操作現代 C++ 字串的 C++ 庫,而 string.h
是一個用於操作 C 樣式字串(即以空字元結尾的字串)的 C 標頭檔案。
讓我們看看下面的程式碼來理解 string.h
庫。
#include <string.h>
#include <iostream>
using namespace std;
int main() {
char country[] = "Pakistan";
char countryTemp[50] = "abc";
cout << "countryTemp length before initializing is:";
cout << strlen(countryTemp) << endl;
// strcpy()
cout << "Let's copy country to countryTemp" << endl;
strcpy(countryTemp, country);
cout << "countryTemp=" << countryTemp << endl;
cout << "countryTemp length after copying country is:";
cout << strlen(countryTemp) << endl;
return 0;
}
上面的程式首先宣告瞭兩個字元陣列(C-strings)並用 Pakistan
初始化第一個。第 10 行列印 countryTemp
的長度,即 3。
雖然 countryTemp
的總大小為 50 個位元組,但 strcpy
只能根據 NULL
字元(放置在陣列的第四個位元組)計算大小。因此,strcpy
返回 3。
第 14 行使用 strcpy
函式將 country
陣列的內容複製到 countryTemp
。因此,countryTemp
的新長度變為 8
。
輸出:
countryTemp length before initializing is:3
Let's copy country to countryTemp
countryTemp=Pakistan
countryTemp length after copying country is:8
注意:
string.h
標頭檔案為NULL
終止的字串(也稱為 C 樣式字串)提供了許多函式。可以在此處找到更多可用的庫函式。
C++ 中字串與 Char 的比較
人們將字串與字元常量進行比較是一個常見的問題。這實際上是不可能的。
參考下面的程式碼。
#include <iostream>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
char inp;
cin >> inp;
if (inp == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
在這個函式中,我們從使用者那裡做了一個輸入,它是一個 char
變數,在 if
條件下,我們將它與一個用雙引號括起來的字串文字進行比較。此程式碼將生成錯誤:ISO C++ 禁止在指標和整數之間進行比較
錯誤。
建議在 string
變數而不是 char
變數中進行輸入以避免此錯誤。
#include <iostream>
#include <string>
using namespace std;
int main() {
cout << "Do you want to proceed (y or n)?\n";
string ans;
cin >> ans;
if (ans == "y") // error here
cout << "Hello again" << endl;
else
cout << "Good Bye" << endl;
return 0;
}
輸出:
Do you want to proceed (y or n)?
n
Good Bye
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn