在 C 語言中查詢系統主機名
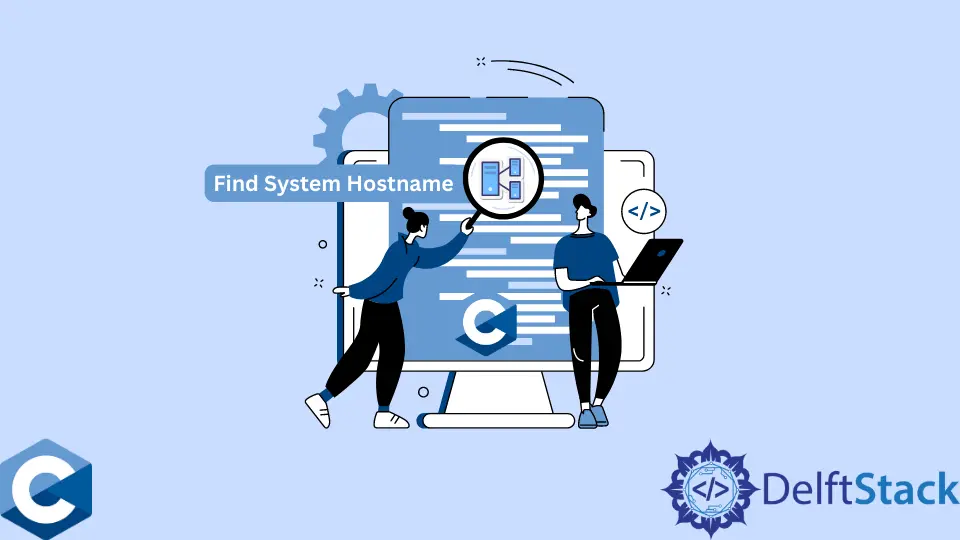
本文將介紹幾種在 C 語言中查詢系統主機名的方法。
使用 gethostname
函式在 C 語言中查詢系統主機名
gethostname
函式是 POSIX 規範的一部分,用於訪問系統主機名。該函式有兩個引數:char*
指向儲存主機名的緩衝區。char*
指向儲存主機名的緩衝區和表示緩衝區長度的位元組數。成功時函式返回 0,錯誤時返回-1。注意 POSIX 系統可能對 hostname 的長度有一個最大的位元組數定義,所以使用者應該分配足夠大的緩衝區來儲存檢索到的值。
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
enum { MAX_SIZE = 256 };
int main(void) {
int ret;
char hostname[MAX_SIZE];
ret = gethostname(&hostname[0], MAX_SIZE);
if (ret == -1) {
perror("gethostname");
exit(EXIT_FAILURE);
}
printf("Hostname: %s\n", hostname);
exit(EXIT_SUCCESS);
}
輸出:
Hostname: lenovo-pc
前面的例子演示了對 gethostname
函式的基本呼叫,但人們應該始終實現錯誤報告例程,以確保故障安全程式碼和資訊豐富的日誌資訊。由於 gethostname
設定了 errno
值,我們可以在 switch
語句中對其進行評估,並將相應的資訊列印到 stderr
流中。
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
enum { MAX_SIZE = 256 };
int main(void) {
int ret;
char hostname[MAX_SIZE];
errno = 0;
ret = gethostname(&hostname[0], MAX_SIZE);
if (ret == -1) {
switch (errno) {
case EFAULT:
fprintf(stderr, "'name' argument is an invalid address");
break;
case EINVAL:
fprintf(stderr, "'len' argument is negative");
break;
default:
perror("gethostname");
}
exit(EXIT_FAILURE);
}
printf("Hostname: %s\n", hostname);
exit(EXIT_SUCCESS);
}
輸出:
Hostname: lenovo-pc
在 C 語言中使用 uname
函式查詢系統主機名
另外,還可以利用 uname
函式呼叫來查詢系統主機名。uname
一般可以檢索到系統的幾個資料點,即作業系統名稱、作業系統釋出日期和版本、硬體識別符號和主機名。但注意,uname
將上述資訊儲存在 <sys/utsname.h>
標頭檔案中定義的 utsntheame
結構中。該函式接收指向 utsname
結構的指標,並在呼叫成功後返回零值。uname
也會在失敗時設定 errno
,當傳遞給 utsname
結構的指標無效時,定義 EFAULT
值作為指示。
#include <stdio.h>
#include <stdlib.h>
#include <sys/utsname.h>
enum { MAX_SIZE = 256 };
int main(void) {
struct utsname uts;
if (uname(&uts) == -1) perror("uname");
printf("Node name: %s\n", uts.nodename);
printf("System name: %s\n", uts.sysname);
printf("Release: %s\n", uts.release);
printf("Version: %s\n", uts.version);
printf("Machine: %s\n", uts.machine);
exit(EXIT_SUCCESS);
}
輸出:
Hostname: lenovo-pc
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn