在 C 語言中初始化結構體
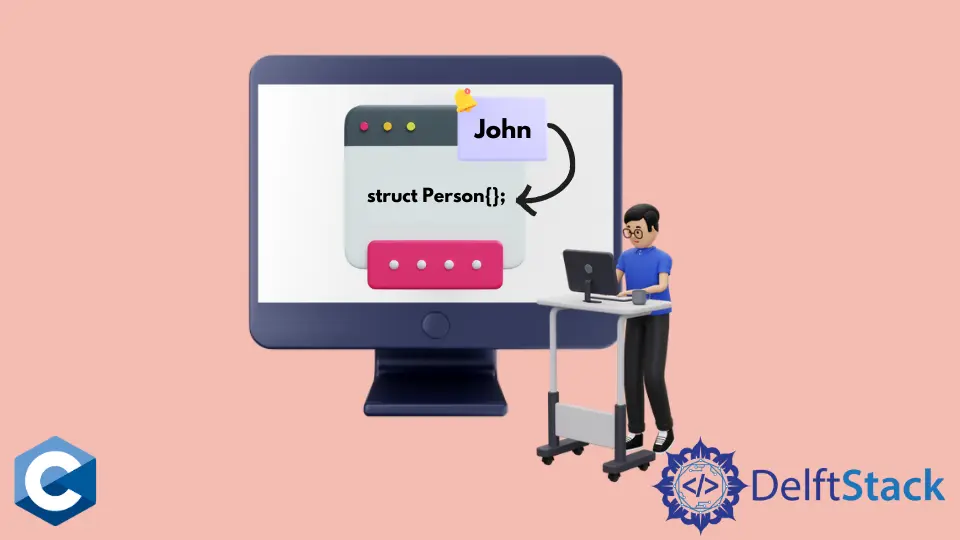
本文將介紹關於如何在 C 語言中初始化一個結構體的多種方法。
使用初始化列表風格的符號來初始化 C 語言中的一個結構體
結構體 struct
可能是 C 語言中構建複雜資料結構最重要的關鍵字,它是一個內建的物件,可以儲存多個異構元素,稱為 members
。
請注意,結構體的定義只用 struct
關鍵字,但在下面的例子中,我們新增 typedef
來建立一個新的型別名,並使後續的宣告更易讀。
一旦定義了結構,我們就可以宣告一個該型別的變數,並用列表符號初始化它。這種語法類似於 C++ 中使用的初始化列表。在這種情況下,我們用一個顯式賦值運算子對 struct
的每個成員進行賦值,但我們只能按照正確的順序指定值,在現代版本的語言中這就足夠了。
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Person {
char firstname[40];
char lastname[40];
int age;
bool alive;
} Person;
int main(void) {
Person me = {
.firstname = "John\0", .lastname = "McCarthy\0", .age = 24, .alive = 1};
printf("Name: %s\nLast Name: %s\nAge: %d\nAlive: ", me.firstname, me.lastname,
me.age);
me.alive ? printf("Yes\n") : printf("No\n");
exit(EXIT_SUCCESS);
}
輸出:
Name: John
Last Name: McCarthy
Age: 24
Alive: Yes
使用賦值列表符號來初始化 C 語言中的結構體
另外,可能會出現這樣的情況,即宣告的 struct
並沒有立即初始化,而是需要在以後的程式中進行賦值。在這種情況下,我們應該使用帶有附加強制轉換符號的初始化程式列表樣式語法作為字首。對 struct
的型別進行轉換是編譯程式的必要步驟。
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Person {
char firstname[40];
char lastname[40];
int age;
bool alive;
} Person;
int main(void) {
Person me;
me = (Person){
.firstname = "John\0", .lastname = "McCarthy\0", .age = 24, .alive = 1};
printf("Name: %s\nLast Name: %s\nAge: %d\nAlive: ", me.firstname, me.lastname,
me.age);
me.alive ? printf("Yes\n") : printf("No\n");
exit(EXIT_SUCCESS);
}
輸出:
Name: John
Last Name: McCarthy
Age: 24
Alive: Yes
在 C 語言中使用個別賦值來初始化結構體
另一種初始化 struct
成員的方法是宣告一個變數,然後分別給每個成員分配相應的值。注意,char
陣列不能用字串賦值,所以需要用額外的函式,如 memcpy
或 memmove
顯式複製(見手冊)。你應該始終注意 array
的長度不能小於被儲存的字串。
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Person {
char firstname[40];
char lastname[40];
int age;
bool alive;
} Person;
int main(void) {
Person me2;
memcpy(&me2.firstname, "Jane\0", 40);
memcpy(&me2.lastname, "Delaney\0", 40);
me2.age = 27;
me2.alive = true;
printf("Name: %s\nLast Name: %s\nAge: %d\nAlive: ", me2.firstname,
me2.lastname, me2.age);
me2.alive ? printf("Yes\n") : printf("No\n");
exit(EXIT_SUCCESS);
}
輸出:
Name: Jane
Last Name: Delaney
Age: 27
Alive: Yes
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn