Angular then() 方法
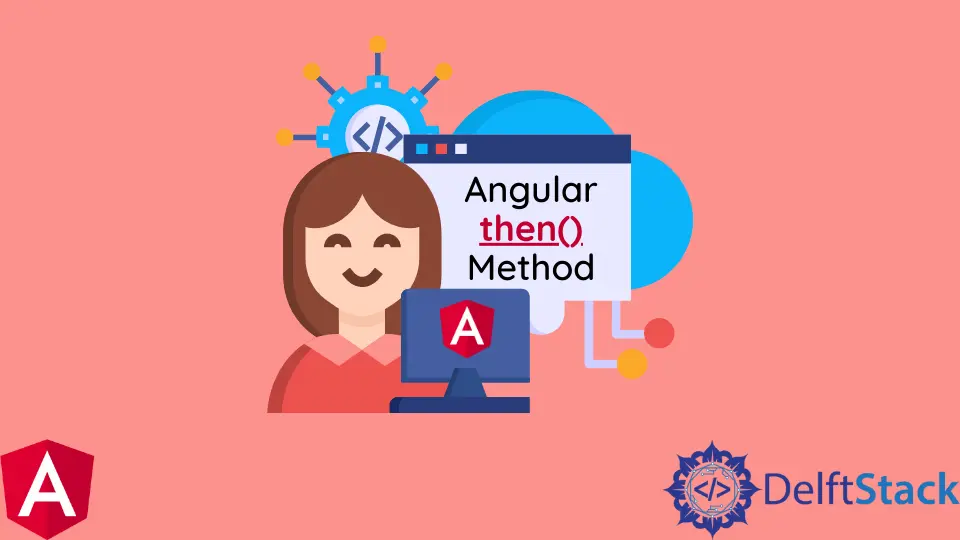
我們將介紹 then()
方法並討論 Angular 中的 promises
。
在 Angular 中使用 then()
方法
then()
是用作第二個引數的 Angular promise
方法。then()
方法僅在 promise
被拒絕時執行。
要詳細瞭解 then()
方法,我們首先必須瞭解 Angular 中的 promises
。
Angular 中的 promise
當應用程式更簡單時,回撥函式會立即被呼叫,但隨著應用程式變得複雜且使用現代瀏覽器的 JavaScript 變得更豐富,回撥變得很麻煩。回撥函式變得更慢,有時在請求資料時會卡住。
作為 ES6
中的解決方案,引入了 promises
來解決問題並簡化它們。它們是用於更好地編寫非同步函式並且易於維護的強大抽象。
promise
是一種 API 抽象,主要用於同步處理非同步操作。
使用以下命令,讓我們建立一個新應用程式。
# angular
ng new my-app
在 Angular 中建立我們的新應用程式後,我們將轉到我們的應用程式目錄。
# angular
cd my-app
現在,讓我們執行我們的應用程式來檢查所有依賴項是否安裝正確。
# angular
ng serve --open
接下來,我們建立一個 promise
,它將在等待 1 秒後返回一個值。首先,我們將匯入 HttpClientModule
並將其新增到 app.module.ts
中 AppModule
的匯入陣列中,如下所示。
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [ BrowserModule, FormsModule, HttpClientModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
然後,我們開始在 app.component.ts
中注入 HttpClient
並建立一個建構函式並定義我們的 API URL。
# angular
import { Component, VERSION, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
name = 'Angular ' + VERSION.major;
apiUrl = 'https://jsonplaceholder.typicode.com/todos/1';
constructor(private httpClient: HttpClient){}
}
一旦我們注入了 HttpClient
,我們定義了一個 fetchJson()
並從 ngOnInit()
呼叫它,所以它在應用程式載入時被初始化。如下圖所示,我們在 app.component.ts
中的程式碼會有額外的程式碼。
# angular
ngOnInit(){
this.fetchJson();
}
private fetchJson(){}
我們將使用 get()
方法傳送 GET HTTP
請求以返回可觀察物件。我們將使用 toPromise()
將其轉換為 promise
,我們將使用 then()
方法。
# angular
private fetchJson(){
const promise = this.httpClient.get(this.apiUrl).toPromise();
console.log(promise);
promise.then((data)=>{
console.log("Promise resolved with: " + JSON.stringify(data));
}).catch((error)=>{
console.log("Promise rejected with " + JSON.stringify(error));
});
}
輸出:
從上面的輸出中,promise
已解決,它還使用 promise 獲取的 then()
方法返回資料。我們可以使用帶有 Promise 的 then()
方法作為第二個引數。
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn