Angular 中的會話儲存
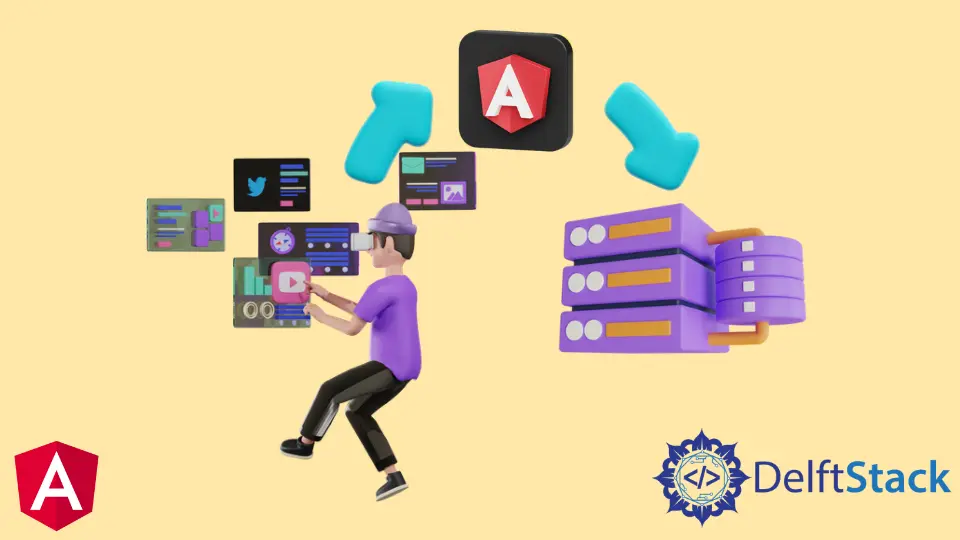
我們將瞭解 sessionStorage
以及如何在 Angular 中的 sessionStorage
中儲存、獲取、刪除特定資料或刪除所有資料。
Angular 中的會話儲存
sessionStorage
為頁面會話期間可用的每個給定源保留一個單獨的儲存區域。sessionStorage
在標籤頁或視窗關閉時重新整理。
使用 sessionStorage
儲存資料
為了在 sessionStorage
中儲存資料,我們將在 app.component.ts
檔案中建立一個函式 saveData()
。
# angular
saveData(){
}
在 saveData
函式中,我們將使用 setItem
將名稱儲存在 sessionStorage
中。
# angular
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
}
我們在 sessionStorage
中儲存資料的功能已經完成。
我們需要編輯我們的 app.component.html
檔案並將帶有 click
事件的按鈕新增到 saveData()
函式。因此,我們在 app.component.html
中的程式碼將如下所示。
# angular
<hello name="{{ name }}"></hello>
<button (click)="saveData()">Save Data in Session Storage</button>
輸出:
當我們點選按鈕時,它將使用 name
的 key
將資料儲存在 sessionStorage
中。
輸出:
從 sessionStorage
獲取資料
我們將討論如何獲取 sessionStorage
中儲存的資料並顯示它。
我們將在 app.component.ts
中建立一個 getData()
函式。
# angular
getData(){
}
在 getData()
函式中,我們將使用 getItem
根據 sessionStorage
中的 key
獲取我們想要的資料。
# angular
getData(){
return sessionStorage.getItem('name');
}
我們現在完成了我們的函式,我們需要顯示從 getData()
函式獲得的資料。
我們將編輯 app.component.html
並替換 hello
標記的 name
屬性。所以我們在 app.component.html
中的程式碼將如下所示。
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()">Save Data in Session Storage</button>
輸出:
從 sessionStorage
中刪除特定資料
我們將討論在單擊按鈕時從 sessionStorage
中刪除基於 key
的特定資料。
首先,我們將在 app.component.ts
中建立 removeData()
函式。
# angular
removeData(){
}
我們將使用 removeItem
根據 key
從 sessionStorage
中刪除特定資料。我們還將在 sessionStorage
中新增一些額外的資料,我們可以在本示例中將其刪除。
app.component.ts
中的程式碼如下所示。
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'Session Storage in Angular 12 By Husnain';
name = 'Angular ' + VERSION.major;
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
sessionStorage.setItem('location', 'Pakistan');
}
getData() {
return sessionStorage.getItem('name');
}
removeData() {
sessionStorage.removeItem('location');
}
}
在上面的程式碼中,我們在 sessionStorage
中新增了帶有 key
位置的額外資料,在按鈕單擊時刪除。
我們需要在 app.component.html
中建立一個按鈕,它將呼叫我們的 removeData()
函式。所以 app.component.html
看起來像這樣。
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
我們還將設定按鈕樣式以使其看起來更好,並在 app.component.css
中新增以下程式碼。
# angular
p {
font-family: Lato;
}
.btn{
margin-right: 15px;
padding: 10px 15px;
background-color: blueviolet;
color: white;
border: none;
border-radius: 5px;
}
輸出:
我們將單擊 Save Data
按鈕以儲存 sessionStorage
位置。之後,我們將單擊 Remove Location
按鈕以從 sessionStorage
中刪除位置資料。
從 sessionStorage
中刪除所有資料
我們將討論從 Angular 中的 sessionStorage
中刪除所有資料。
我們將在 app.component.ts
中建立 deleteData()
函式。
# angular
deleteData(){
}
我們將使用 clear
刪除 sessionStorage
中的所有資料。所以我們的程式碼如下所示。
# angular
deleteData(){
sessionStorage.clear();
}
我們需要在 app.component.html
中建立一個按鈕來呼叫 deleteData()
函式。所以我們的程式碼如下所示。
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
<button (click)="deleteData()" class="btn">Clear All</button>
輸出:
最後結果:
我們的檔案最終將如下所示。
app.component.ts
:
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'Session Storage in Angular 12 By Husnain';
name = 'Angular ' + VERSION.major;
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
sessionStorage.setItem('location', 'Pakistan');
}
getData() {
return sessionStorage.getItem('name');
}
removeData() {
sessionStorage.removeItem('location');
}
deleteData() {
sessionStorage.clear();
}
}
app.component.html
:
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
<button (click)="deleteData()" class="btn">Clear All</button>
app.component.css
:
# angular
p {
font-family: Lato;
}
.btn {
margin-right: 15px;
padding: 10px 15px;
background-color: blueviolet;
color: white;
border: none;
border-radius: 5px;
}
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn