在 Angular 的下拉選單中選擇一個值
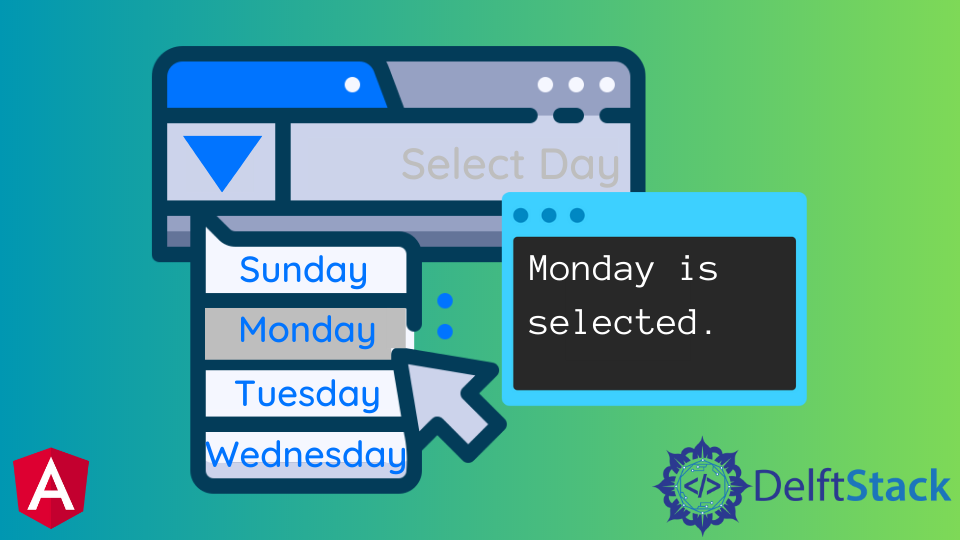
在 Angular 2 中,ngOptions
指令用於建立下拉選單,ngFor
指令用於迭代下拉值,ngif
用於選擇一個值。本文將演示如何在 Angular 中選擇下拉選單。
在 Angular 2 中的下拉選單中選擇值的步驟
從下拉選單中選擇一個值是 Web 應用程式中最常見的任務之一。
在 Angular 2 的下拉選單中選擇一個值需要以下步驟。
-
開啟程式碼編輯器。
-
建立一個下拉選單。
-
新增按鈕以開啟下拉選單以新增專案。
-
新增一個帶有
ng-for
指令的空div
元素,以表格格式顯示所有專案。 -
新增一個按鈕,該按鈕將呼叫函式以在單擊時關閉並儲存在此檢視中所做的更改。
-
新增
ngif
指令以選擇正確的選項。
讓我們編寫一個示例來應用上述步驟。
TypeScript 程式碼(App.component.ts
):
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
selectedOption = 0;
actions = [{ id: 0, name: 'Select Country' },
{ id: 1, name: 'Netherland' },
{ id: 2, name: 'England' },
{ id: 3, name: 'Scotland' },
{ id: 4, name: 'Germany' },
{ id: 5, name: 'Canada' },
{ id: 6, name: 'Mexico' },
{ id: 7, name: 'Spain' }]
}
選擇器和模板是 @Component
後設資料物件的兩個欄位。選擇器欄位為代表元件的 HTML 元素指定選擇器。
該模板指示 Angular 如何顯示該元件的檢視。你可以將 URL 新增到 HTML 檔案或將 HTML 放在這裡。
在此示例中,我們使用 URL 指向 HTML 模板。之後,我們編寫了要在輸出終端中顯示的選項。
TypeScript 程式碼:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
@NgModule
定義了模組的後設資料。BrowserModule
跟蹤重要的應用服務提供商。
它還包括標準指令,如 ngIf
和 ngFor
,它們會立即出現並可以在任何模組的元件模板中使用。ngModel
指令需要 FormsModule
模組。
宣告列表指定應用程式元素和根元素,它位於應用程式元件層次結構的開頭。宣告陣列包含模組、命令和管道的列表。
HTML 程式碼(App.component.html
):
<h2>Example of how to select the value in the dropdown list in Angular 2</h2>
<select id="select-menu" [(ngModel)]="selectedOption" class="bx--text-input" required name="actionSelection" >
<option *ngFor="let action of actions" [value]="action.id">{{action.name}}</option>
</select>
<div>
<div *ngIf="selectedOption==1">
<div>Netherland is selected</div>
</div>
<div *ngIf="selectedOption==2">
<div>England is selected</div>
</div>
<div *ngIf="selectedOption==3">
<div>Scotland is selected</div>
</div>
<div *ngIf="selectedOption==4">
<div>Germany is selected</div>
</div>
<div *ngIf="selectedOption==5">
<div>Canada is selected</div>
</div>
<div *ngIf="selectedOption==6">
<div>Mexico is selected</div>
</div>
<div *ngIf="selectedOption==7">
<div>Spain is selected</div>
</div>
</div>
在這段程式碼中,我們使用了兩個指令,ngfor
和 ngif
。
ngfor
指令用於建立專案列表。這可以是一個簡單的陣列,也可以是一個將某些屬性轉換為陣列的物件。
ngfor
指令通常用於遍歷陣列併為列表中的每個專案呈現不同的 DOM 元素。在這裡,ngfor
的目的是建立一個國家列表。
其次,我們使用了 ngif
,這是 Angular 中建立 if-else
語句的指令。它也可以與 ngElse
、ngSwitch
和 ngShow/ngHide
一起使用。
該指令僅在表示式計算結果為 true 時呈現 HTML 程式碼。如果計算結果為 false,則不會渲染任何內容。
在這裡,ngif
只會顯示所選國家。
點選這裡檢視上面程式碼的演示。
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook