Angular 中的資料表
Rana Hasnain Khan
2023年1月30日
2022年4月20日
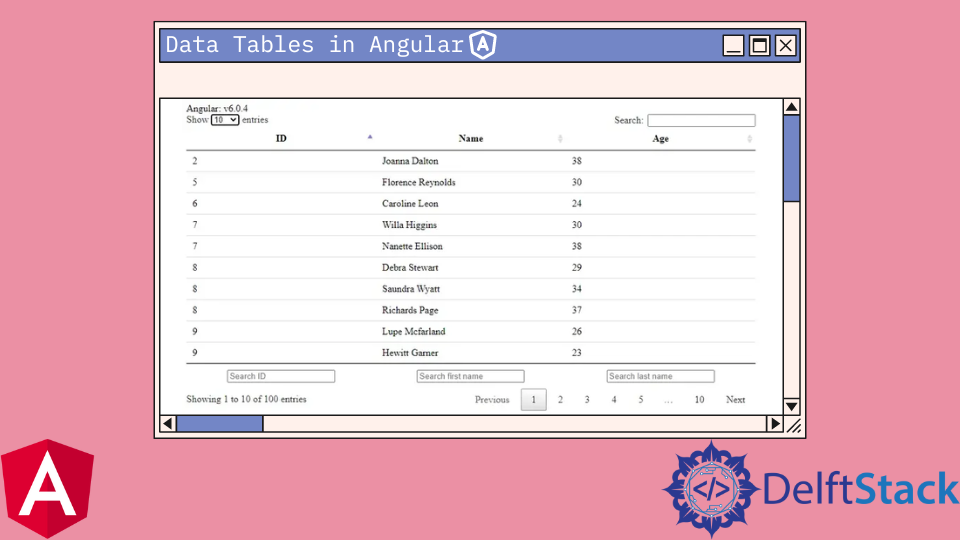
我們將介紹如何使用資料表並將 JSON 響應轉換為 Angular 中的資料表。
Angular 中的資料表
有一個預建的 angular-datatables
庫,我們可以使用它在 Angular 中顯示覆雜的資料表。
我們可以使用以下命令輕鬆安裝它:
# terminal
ng add angular-datatables
或者我們可以使用 npm
手動安裝它:
# terminal
npm install jquery --save
npm install datatables.net --save
npm install datatables.net-dt --save
npm install angular-datatables --save
npm install @types/jquery --save-dev
npm install @types/datatables.net --save-dev
之後,我們需要將指令碼和樣式屬性中的依賴項新增到 angular.json
檔案中。
# angular
"projects": {
"your-app-name": {
"architect": {
"build": {
"options": {
"styles": [
"node_modules/datatables.net-dt/css/jquery.dataTables.css"
],
"scripts": [
"node_modules/jquery/dist/jquery.js",
"node_modules/datatables.net/js/jquery.dataTables.js"
],
...
}
}
現在,我們需要在應用程式的適當級別匯入 DataTablesModule
。
# angular
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { DataTablesModule } from "angular-datatables";
import { AppComponent } from "./app.component";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, DataTablesModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
在 Angular 中將 JSON 轉換為資料表
如果我們想將 JSON 響應轉換為 DataTables
,我們可以使用以下示例。
我們需要將以下程式碼新增到 app.component.ts
。
# angular
import { AfterViewInit, VERSION, Component, OnInit, ViewChild } from '@angular/core';
import { DataTableDirective } from 'angular-datatables';
import { Subject } from 'rxjs';
import 'rxjs/add/operator/map';
import { HttpParams, HttpClient, HttpHeaders } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
})
export class AppComponent implements OnInit, AfterViewInit {
version = 'Angular: v' + VERSION.full;
@ViewChild(DataTableDirective)
datatableElement: DataTableDirective;
dtOptions: DataTables.Settings = {};
persons: any = [];
// We use this trigger because fetching the list of persons can be quite long,
// thus we make sure the data gets fetched before rendering
dtTrigger: Subject<any> = new Subject();
constructor(private http: HttpClient) { }
ngOnInit(): void {
const dataUrl = 'https://raw.githubusercontent.com/Inventico-Sol/test-json/main/data.json';
this.http.get(dataUrl)
.subscribe(response => {
setTimeout(() => {
this.persons = response.data;
console.log(response);
// Calling the DT trigger to manually render the table
this.dtTrigger.next();
});
});
}
ngAfterViewInit(): void {
this.datatableElement.dtInstance.then((dtInstance: DataTables.Api) => {
dtInstance.columns().every(function () {
const that = this;
$('input', this.footer()).on('keyup change', function () {
if (that.search() !== this['value']) {
that
.search(this['value'])
.draw();
}
});
});
});
}
}
然後,我們必須將以下程式碼新增到 app.component.html
。
# angular
{{ version }}
<table
datatable
[dtOptions]="dtOptions"
[dtTrigger]="dtTrigger"
class="row-border hover"
>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let person of persons">
<td>{{ person.id }}</td>
<td>{{ person.name }}</td>
<td>{{ person.age }}</td>
</tr>
</tbody>
<tfoot>
<tr>
<th><input type="text" placeholder="Search ID" name="search-id" /></th>
<th>
<input
type="text"
placeholder="Search first name"
name="search-first-name"
/>
</th>
<th>
<input
type="text"
placeholder="Search last name"
name="search-last-name"
/>
</th>
</tr>
</tfoot>
</table>
輸出:
Author: Rana Hasnain Khan
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn