Angular 2 模態對話方塊
- 什麼是 Angular 2 中的模態對話方塊
- 匯入庫以在 Angular 2 中建立模態對話方塊
- 在 Angular 2 中建立模態對話方塊的模態服務
- 自定義模態元件以在 Angular 2 中建立模態對話方塊
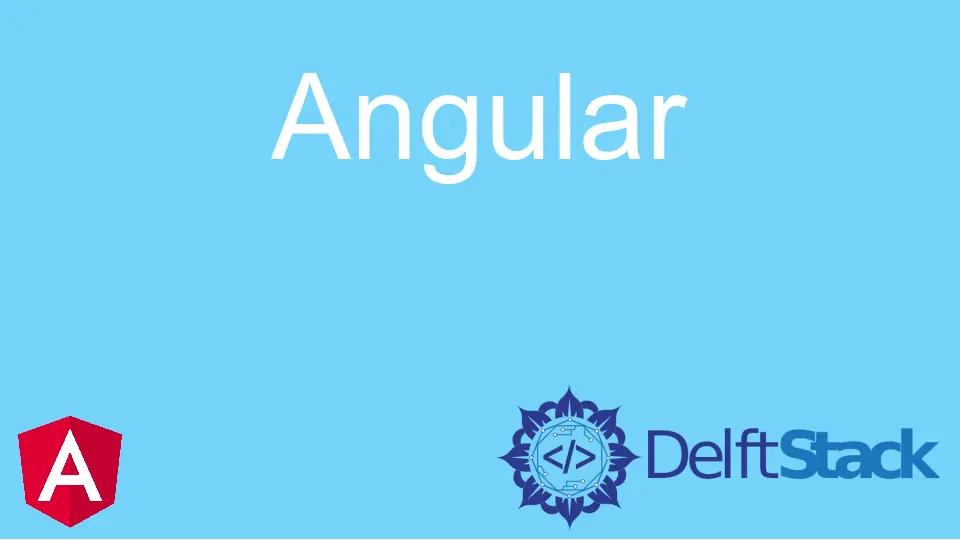
Angular 2.0 是流行的 JavaScript 框架的最新版本。它為開發人員提供了一種新的基於元件的架構,支援移動裝置,並且是用 TypeScript 編寫的。
模態對話方塊是在當前視窗頂部彈出並阻止互動直到關閉的視窗。本文將詳細解釋 Angular 2.0 模態對話方塊。
什麼是 Angular 2 中的模態對話方塊
為了幫助開發人員建立更具動態性和互動性的 Web 應用程式,Angular 2.0 引入了一個名為 Modal Dialog
的新元件。
術語 modal dialog
和 modal window
可能看起來令人困惑,但事實並非如此。大多數人預設將模態視窗
稱為彈出視窗。
模態對話方塊
使開發人員能夠建立具有豐富內容和動畫的各種對話方塊。它可以以不同的方式使用,例如:
- 通過要求輸入來提示使用者。
- 顯示重要訊息或通知。
- 顯示錯誤資訊或確認資訊。
點選這裡如果你想了解更多關於模態對話方塊的資訊。
為簡單起見,讓我們將工作分為以下幾部分。
- 新增庫
- 模態服務
- 模態元件
出於造型目的,我們使用了 Bootstrap。
匯入庫以在 Angular 2 中建立模態對話方塊
在 index.html
檔案中匯入以下庫以增強程式碼的功能。
<script src="https://npmcdn.com/core-js/client/shim.min.js"></script>
<link href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css" rel="stylesheet">
<script src="https://npmcdn.com/zone.js@0.6.12?main=browser"></script>
<script src="https://npmcdn.com/reflect-metadata@0.1.3"></script>
<script src="https://npmcdn.com/systemjs@0.19.27/dist/system.src.js"></script>
在此之後,我們主要關心的是編譯程式碼。你不能用 Angular 2 直接將東西編譯到 DOM;你需要一個佔位符。
這就是我們製作 modal placeholder
的原因。請記住,這是開始的最重要步驟。
@Component({
selector: "modal-placeholder",
template: `<div #modalplaceholder></div>`
})
export class ModalPlaceholderComponent implements OnInit {
@ViewChild("modalplaceholder", {read: ViewContainerRef}) viewContainerRef;
在 Angular 2 中建立模態對話方塊的模態服務
我們正在邁向第二步,也稱為根步。模態服務
的主要目的是使頁面和模態元件更容易通訊。
模態服務還跟蹤哪些頁面模態可用以及如何與它們互動。
import {Component,NgModule, ViewChild, OnInit, ViewContainerRef, Compiler, ReflectiveInjector, Injectable, Injector, ComponentRef} from "@angular/core";
import {Observable, Subject, BehaviorSubject, ReplaySubject} from "rxjs/Rx";
@Injectable()
export class ModalService {
private vcRef: ViewContainerRef;
private injector: Injector;
public activeInstances: number;
constructor(private compiler: Compiler) {
}
registerViewContainerRef(vcRef: ViewContainerRef): void {
this.vcRef = vcRef;
}
registerInjector(injector: Injector): void {
this.injector = injector;
}
create<T>(module: any, component: any, parameters?: Object): Observable<ComponentRef<T>> {
let componentRef$ = new ReplaySubject();
this.compiler.compileModuleAndAllComponentsAsync(module)
.then(factory => {
let componentFactory = factory.componentFactories.filter(item => item.componentType === component)[0];
const childInjector = ReflectiveInjector.resolveAndCreate([], this.injector);
let componentRef = this.vcRef.createComponent(componentFactory, 0, childInjector);
Object.assign(componentRef.instance, parameters);
this.activeInstances ++;
componentRef.instance["com"] = this.activeInstances;
componentRef.instance["destroy"] = () => {
this.activeInstances --;
componentRef.destroy();
};
componentRef$.next(componentRef);
componentRef$.complete();
});
return <Observable<ComponentRef<T>>> componentRef$.asObservable();
}
}
@Component({
selector: "modal-placeholder",
template: `<div #modalplaceholder></div>`
})
export class ModalPlaceholderComponent implements OnInit {
@ViewChild("modalplaceholder", {read: ViewContainerRef}) viewContainerRef;
constructor(private modalService: ModalService, private injector: Injector) {
}
ngOnInit(): void {
this.modalService.registerViewContainerRef(this.viewContainerRef);
this.modalService.registerInjector(this.injector);
}
}
@NgModule({
declarations: [ModalPlaceholderComponent],
exports: [ModalPlaceholderComponent],
providers: [ModalService]
})
export class ModalModule {
}
export class ModalContainer {
destroy: Function;
componentIndex: number;
closeModal(): void {
this.destroy();
}
}
export function Modal() {
return function (world) {
Object.assign(world.prototype, ModalContainer.prototype);
};
}
什麼是 RxJS
RxJS
(JavaScript 的響應式擴充套件)是一套模組,允許你使用可見陣列和組合在 JavaScript 中建立非同步和基於事件的程式。
自定義模態元件以在 Angular 2 中建立模態對話方塊
自定義模態指令可以使用 <modal>
標籤在 Angular 應用程式中新增模態。
當一個模態例項載入時,它會向 ModalService
註冊,以便該服務可以開啟和關閉模態視窗。當使用 Destroy
方法銷燬時,它會從 ModalService
登出。
import {Modal} from "./modal.module";
import {Component} from "@angular/core";
@Component({
selector: "my-cust",
template: `
<h1>Basic Components of a Car</h1>
<button (click)="onDelete()">×</button>
<ul>
<li *ngFor="let option of options">{{option}}</li>
</ul>
<div>
<button (click)="onDelete()">
<span>Delete</span>
</button>
<button (click)="onSave()">
<span>Save</span>
</button>
</div>
`
})
@Modal()
export class ModalComponent {
ok: Function;
destroy: Function;
closeModal: Function;
options = ["Speed", "Mileage", "Color"];
onDelete(): void{
this.closeModal();
this.destroy();
}
onSave(): void{
this.closeModal();
this.destroy();
this.ok(this.options);
}
}
最後,index.html
程式碼如下。
<!DOCTYPE html>
<html>
<head>
<title>Angular 2 QuickStart</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- 1. Load libraries -->
<!-- Polyfill(s) for older browsers -->
<script src="https://npmcdn.com/core-js/client/shim.min.js"></script>
<link href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css" rel="stylesheet">
<script src="https://npmcdn.com/zone.js@0.6.12?main=browser"></script>
<script src="https://npmcdn.com/reflect-metadata@0.1.3"></script>
<script src="https://npmcdn.com/systemjs@0.19.27/dist/system.src.js"></script>
<!-- 2. Configure SystemJS -->
<script src="config.js"></script>
<script>
System.import('app').catch(function(err){ console.error(err); });
</script>
</head>
<body>
<my-hoop>Loading...</my-hoop>
</body>
</html>
因此,這就是在 Angular 2 中建立模態的方式。程式碼可維護、靈活且易於使用。點選這裡檢視程式碼的演示。
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook