Pandas Series Series.map() 功能
Minahil Noor
2023年1月30日
-
pandas.Series.map()
語法 -
示例程式碼:
Series.map()
-
示例程式碼:
Series.map()
傳遞一個字典作為arg
引數 -
示例程式碼:
Series.map()
傳遞一個函式作為arg
引數 -
示例程式碼:
Series.map()
應用於DataFrame
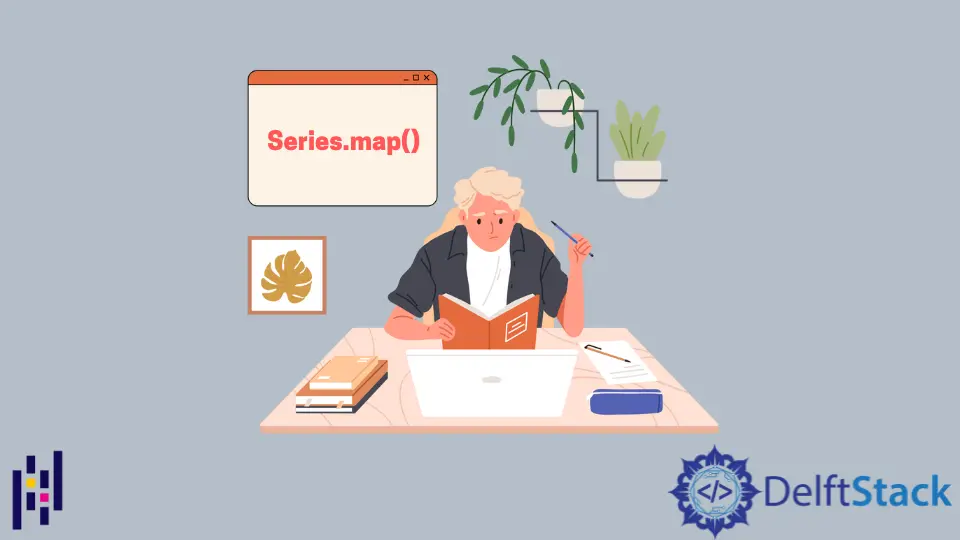
Python Pandas Series.map()
函式替換一個 Series
的值。被替換的值可能來自於 Series
、字典或一個函式。這個函式只對一個 Series
有效。如果我們將此函式應用於 DataFrame
,那麼它將產生一個 AttributeError
。
pandas.Series.map()
語法
Series.map(arg, na_action=None)
引數
arg |
它是函式,字典或 Series 。要替換的值來自這個函式、字典或 Series 。 |
na_action |
該引數接受兩個值。None 和 ignore 。它的預設值是 None 。如果它的值是 ignore ,那麼它就不會將派生值對映到 NaN 值。它忽略 NaN 值。 |
返回值
它返回一個與呼叫者具有相同索引的 Series
。
示例程式碼:Series.map()
我們將生成一個包含 NaN
值的 Series
,以檢查傳遞 na_action
引數後的輸出。
import pandas as pd
import numpy as np
series = pd.Series(['Rose',
'Lili',
'Tulip',
np.NaN,
'Orchid',
'Hibiscus',
'Jasmine',
'Daffodil',
np.NaN ,
'SunFlower',
'Daisy'])
print(series)
示例 Series
是:
0 Rose
1 Lili
2 Tulip
3 NaN
4 Orchid
5 Hibiscus
6 Jasmine
7 Daffodil
8 NaN
9 SunFlower
10 Daisy
dtype: object
我們使用 NumPy
來生成 NaN
值。
引數 arg
是一個強制性引數。如果它沒有被傳遞,那麼函式會產生一個 TypeError
。我們將首先傳遞一個 Series 作為 arg
引數。
為了對映兩個 Series,第一個 Series 的最後一列應該與第二個 Series 的索引相同。
import pandas as pd
import numpy as np
first_series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
second_series = pd.Series(
[23, 34, 67, 90, 21, 45, 29, 70, 56],
index=[
"Rose",
"Lili",
"Tulip",
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
"SunFlower",
"Daisy",
],
)
series1 = first_series.map(second_series)
print(series1)
輸出:
0 23.0
1 34.0
2 67.0
3 NaN
4 90.0
5 21.0
6 45.0
7 29.0
8 NaN
9 70.0
10 56.0
dtype: float64
請注意,函式在比較了兩個 Series 之後,已經替換了這些值。
示例程式碼:Series.map()
傳遞一個字典作為 arg
引數
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
dictionary = {
"Rose": "One",
"Lili": "Two",
"Orchid": "Three",
"Jasmine": "Four",
"Daisy": "Five",
}
series1 = series.map(dictionary)
print(series1)
輸出:
0 One
1 Two
2 NaN
3 NaN
4 Three
5 NaN
6 Four
7 NaN
8 NaN
9 NaN
10 Five
dtype: object
Series
中不在字典中的值會被 NaN
值代替。
示例程式碼:Series.map()
傳遞一個函式作為 arg
引數
現在我們將傳遞一個函式作為引數。
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format)
print(series1)
輸出:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 The name of the flower is nan.
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 The name of the flower is nan.
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
這裡,我們傳遞了 string.format()
函式作為引數。請注意,該函式也被應用於 NaN
值。如果我們不想將該函式應用於 NaN 值,那麼我們將把 ignore
值傳遞給 na_action
引數。
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format, na_action="ignore")
print(series1)
輸出:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 NaN
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 NaN
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
上面的示例程式碼已經忽略了 NaN
值。
示例程式碼:Series.map()
應用於 DataFrame
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.map("The flower name is {}.".format)
print(dataframe1)
輸出:
AttributeError: 'DataFrame' object has no attribute 'map'
函式產生了 AttributeError
。