Pandas DataFrame DataFrame.set_index() 函式
Suraj Joshi
2023年1月30日
-
pandas.DataFrame.set_index()
方法的語法 -
示例程式碼:用 Pandas
DataFrame.set_index()
方法設定 Pandas DataFrame 索引 -
示例程式碼:在 Pandas
DataFrame.set_index()
方法中設定drop=False
-
示例程式碼在 Pandas
DataFrame.set_index
方法中設定inplace=True
-
示例程式碼:使用 Pandas
DataFrame.set_index()
方法設定多索引列 -
示例程式碼:Pandas
Dataframe.set_index()
在verify_integrity
為True
時的行為
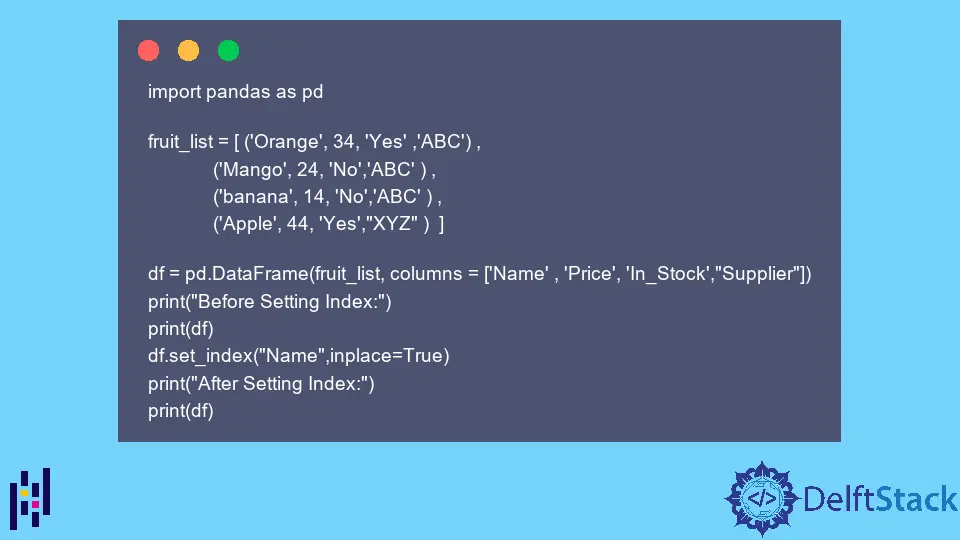
pandas.DataFrame.set_index()
方法可以用來設定適當長度的陣列或列作為 DataFrame 的索引,即使在 DataFrame 建立後也可以使用。新設定的索引可以替代現有的索引,也可以在現有索引的基礎上進行擴充套件。
pandas.DataFrame.set_index()
方法的語法
DataFrame.set_index(
keys, drop=True, append=False, inplace=False, verify_integrity=False
)
引數
keys |
列或列列表作為索引設定 |
drop |
布林型。預設值為 True ,刪除要設定為索引的列 |
append |
布林型。預設值是 False ,它指定是否將列追加到現有的索引中 |
inplace |
布林型。如果 True ,則在原地修改呼叫者的 DataFrame |
verify_integrity |
布林型。如果是 True ,在建立有重複的索引時引發 ValueError 。預設值是 False |
返回值
如果 inplace
為 True
,則返回一個帶有修改過的索引列的 DataFrame
物件;否則為 None
。
示例程式碼:用 Pandas DataFrame.set_index()
方法設定 Pandas DataFrame 索引
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name")
print(df_modified)
輸出:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
4 Pineapple 64 No XYZ
5 Kiwi 84 Yes XYZ
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
Pineapple 64 No XYZ
Kiwi 84 Yes XYZ
原始的 Dataframe
將數字範圍作為預設的索引列,而在 modified_df
中,我們使用 set_index()
方法設定 Name
列作為索引。
示例程式碼:在 Pandas DataFrame.set_index()
方法中設定 drop=False
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name",drop=False)
print(df_modified)
輸出:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
Name Price In_Stock Supplier
Name
Orange Orange 34 Yes ABC
Mango Mango 24 No ABC
banana banana 14 No ABC
Apple Apple 44 Yes XYZ
如果我們在 DataFrame 的 set_index
方法中設定 drop=False
,即使 Name
列被設定為 index
列,它仍然是 Dataframe
中的一列。
示例程式碼在 Pandas DataFrame.set_index
方法中設定 inplace=True
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",inplace=True)
print("After Setting Index:")
print(df)
輸出:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
如果我們在 set_index()
方法中設定 inplace=True
,呼叫者 DataFrame
將被原地修改。
示例程式碼:使用 Pandas DataFrame.set_index()
方法設定多索引列
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",append=True,inplace=True,drop=False)
print("After Setting Index:")
print(df)
輸出:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Name Price In_Stock Supplier
Name
0 Orange Orange 34 Yes ABC
1 Mango Mango 24 No ABC
2 banana banana 14 No ABC
3 Apple Apple 44 Yes XYZ
如果我們在 set_index()
方法中設定 append=True
,則會將新設定的索引列追加到現有的索引中,並且單個 DataFrame
有多個索引列。
示例程式碼:Pandas Dataframe.set_index()
在 verify_integrity
為 True
時的行為
import pandas as pd
fruit_list = [
("Orange", 34, "Yes", "ABC"),
("Mango", 24, "No", "ABC"),
("Apple", 14, "No", "ABC"),
("Apple", 44, "Yes", "XYZ"),
]
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "In_Stock", "Supplier"])
df_modified = df.set_index("Name", verify_integrity=True)
print(df_modified)
輸出:
Traceback (most recent call last):
.....line 3920, in set_index
dup=duplicates))
ValueError: Index has duplicate keys: Index(['Apple'], dtype='object', name='Name')
因為索引有重複的索引- Apple
,所以會引發 ValueError
。它有兩個 Apple
在列中被設定為索引;因此,如果 set_index()
方法中的 verify_integrity
被設定為 True
,它就會引發一個錯誤。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn